Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial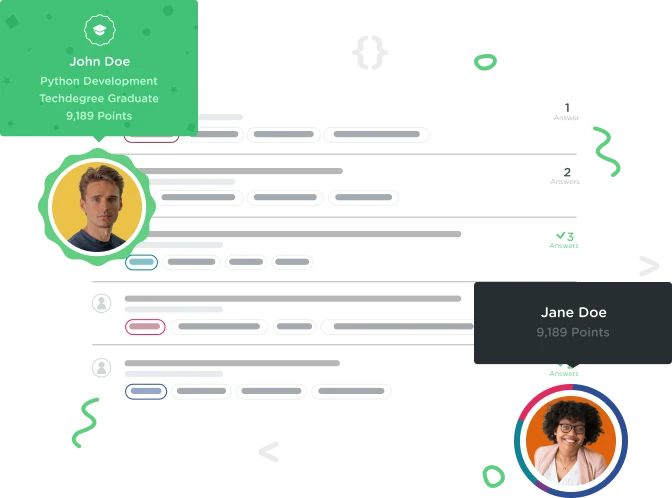
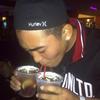
Dean Onizuka
4,753 PointsvalidatedFieldName challenge
not sure why my code isnt working. i get an error message that it doesnt recognize the isUppercase variable. i also tried isUpperCase and that doesnt work either. any suggestions?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (fieldName .charAt(0) != 'm') {
throw new IllegalArgumentException("Error!");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
if (fieldName.charAt(1) != Character.isUppercase) {
throw new IllegalArgumentException("Error!");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (fieldName .charAt(0) != 'm') {
throw new IllegalArgumentException("Error!");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
if (fieldName.charAt(1) != Character.isUppercase) {
throw new IllegalArgumentException("Error!");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
3 Answers
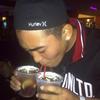
Dean Onizuka
4,753 Pointsupdated code. still not working. error incomparable type char and boolean
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (fieldName .charAt(0) != 'm') {
throw new IllegalArgumentException("Error!");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
if (fieldName.charAt(1) != Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("Error!");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}

Craig Dennis
Treehouse TeacherSomething looks wrong with this line:
if (fieldName .charAt(0) != 'm') {
HINT: Looking at it too long might make you spacey

William Briffa
6,919 PointsWhen calling Character.isUppercase(), you need to supply it with an argument of a character, and it will check to see if the supplied character is uppercase. I believe this should work for you: Character.isUpperCase(fieldName.charAt(1))
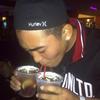
Dean Onizuka
4,753 Pointsstill not working . its calling the != and saying incomparable types... char and boolean
William Briffa
6,919 PointsWilliam Briffa
6,919 PointsYou don't want to compare them with the !=, you only want to check the Character.isUppercaseMethod, that'll return the boolean you're looking for. Here's a better way of putting it: if (Character.isUpperCase(fieldName.charAt(1))) is all you need.
Dean Onizuka
4,753 PointsDean Onizuka
4,753 Pointsbut that above code line says if character is uppercase then it will fail. hmmmmm
William Briffa
6,919 PointsWilliam Briffa
6,919 PointsAh, my fault. A not(!)operator would be necessary. if (!Character.isUpperCase(fieldName.charAt(1))) That should do it!
Dean Onizuka
4,753 PointsDean Onizuka
4,753 Pointsgot it! thanks william
William Briffa
6,919 PointsWilliam Briffa
6,919 PointsSorry it took me a couple times!