Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial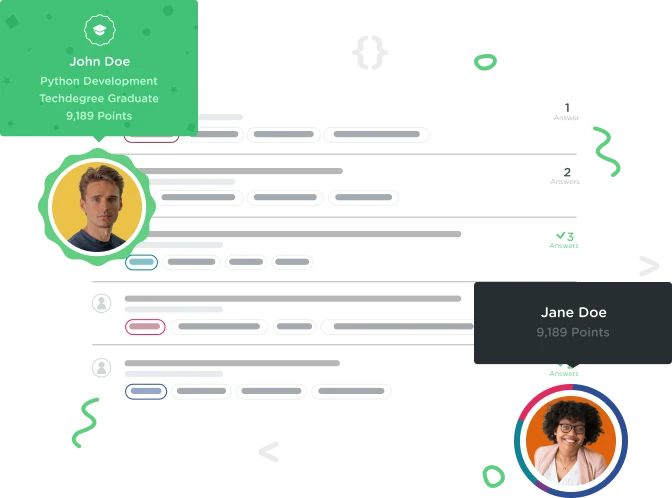

Elizabeth Eisenstein
128 PointsValidating Contact form error message
Hi everyone. I've been following Randy's "Building a Simple PHP Program" lesson, and everything has been going smoothly, except my contact form. I feel like I've probably overlooked something, or there's some obvious, silly error staring me in the face, but I can't find it!
When I test out my contact form, it always gives me the error message that I haven't entered a name, email, or message in the contact form--even when I've entered everything accurately.
I'll attach the code, but I'm pretty sure it's verbatim what was written out in the lessons . Any help is much appreciated!
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = trim($_POST["name"]); $email = trim($_POST["email"]); $message = trim($_POST["message"]);
if ($name == "" OR $email_address == "" OR $message == "") {
echo "You must specify a value for name, email address, and message.";
exit;
}
foreach( $_POST as $value ){
if( stripos($value, 'Content-Type:') !== FALSE ){
echo "There was a problem with the information you entered.";
exit;
}
}
if ($_POST["address"] != "") {
echo "Your form submission has an error.";
exit;
}
require_once("inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)){
echo "You must specify a valid email address.";
exit;
}
$email_body = "";
$email_body = $email_body . "Name: " . $name . "\n";
$email_body = $email_body . "Email: " . $email . "\n";
$email_body = $email_body . "Message: " . $message;
// TODO : send email
header("Location: about.php?status=thanks");
exit;
} ?>
4 Answers
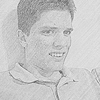
Elliott Frazier
Courses Plus Student 9,647 PointsGot it! You seem to have put a value of "message" in the name attribute of the input
tag that your using for a "honey-pot" validation check instead of a value of "address". that's why it couldn't pass the validation check and kept giving you that error, because the "honey-pot" input's value is blank and since the name attribute was set to "message" it ran that though the first validation check to see if it's value is blank and it was so it gave you the error you told it to :
if ($name == "" OR **$email** == "" OR $message == "") {
echo "You must specify a value for name, email address, and message.";
exit;
}
edit -
I also changed the if
statement above by replacing the varible: "$email_address" to: "$email" because you stored $_POST["email"]
in the varible "$email"
Hope this helps :)
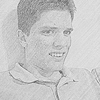
Elliott Frazier
Courses Plus Student 9,647 PointsYou need to show the html aswell.

Elizabeth Eisenstein
128 Points<form method="post" action="about.php">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input type="text" name="name" id="name">
</td>
</tr>
<tr>
<th>
<label for="email">Email</label>
</th>
<td>
<input type="text" name="email" id="email">
</td>
</tr>
<tr>
<th>
<label for="message">Message</label>
</th>
<td>
<textarea name="message" id="message"></textarea>
</td>
</tr>
<tr style="display: none;">
<th>
<label for="address">Address</label>
</th>
<td>
<input type="text" name="message" id="message">
<p>Humans: please leave this field blank!</p>
</td>
</tr>
</table>
<input type="submit" value="send">
</form>
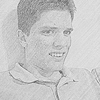
Elliott Frazier
Courses Plus Student 9,647 PointsYou seem to have accidentally cut out the opening form tag. could you display that aswell?

Elizabeth Eisenstein
128 Pointsah sorry, here's everything all together:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = trim($_POST["name"]); $email = trim($_POST["email"]); $message = trim($_POST["message"]);
if ($name == "" OR $email_address == "" OR $message == "") {
echo "You must specify a value for name, email address, and message.";
exit;
}
foreach( $_POST as $value ){
if( stripos($value, 'Content-Type:') !== FALSE ){
echo "There was a problem with the information you entered.";
exit;
}
}
if ($_POST["address"] != "") {
echo "Your form submission has an error.";
exit;
}
require_once("inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)){
echo "You must specify a valid email address.";
exit;
}
$email_body = "";
$email_body = $email_body . "Name: " . $name . "\n";
$email_body = $email_body . "Email: " . $email . "\n";
$email_body = $email_body . "Message: " . $message;
// TODO : send email
header("Location: about.php?status=thanks");
exit;
} ?> <?php $pageTitle = "+ ABOUT"; include('inc/header.php'); ?>
<?php $section = "ABOUT"; include('inc/shopnav.php'); ?>
<!--images should be under 220 wide-->
<div id="shopcontainer" class="container column">
<div id="shopbox" class="thirteen columns clearfix" style="min-height:300px;">
<h3>Contact</h3>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") { ?>
<p>Thank you for your message</p>
<?php } else { ?>
<p>
about about about about
</p>
<form method="post" action="about.php">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input type="text" name="name" id="name">
</td>
</tr>
<tr>
<th>
<label for="email">Email</label>
</th>
<td>
<input type="text" name="email" id="email">
</td>
</tr>
<tr>
<th>
<label for="message">Message</label>
</th>
<td>
<textarea name="message" id="message"></textarea>
</td>
</tr>
<tr style="display: none;">
<th>
<label for="address">Address</label>
</th>
<td>
<input type="text" name="message" id="message">
<p>Humans: please leave this field blank!</p>
</td>
</tr>
</table>
<input type="submit" value="send">
</form>
<?php } ?>
</div>
</div>
<?php include ('inc/footer.php'); ?>

Elizabeth Eisenstein
128 PointsHm, so I changed the name and id attribute of the input tag for the hidden "Address" field...but it still didn't work. I still get my error message of "You must specify a value for name, email address, and message." regardless of what I enter into the form. Did I mistake what you were telling me?
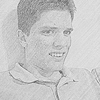
Elliott Frazier
Courses Plus Student 9,647 PointsI edited my previous answer with more information accidentally left out. hope it helps ;)

Elizabeth Eisenstein
128 PointsFantastic! Thank you so much--feel sorta silly for missing that one!