Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial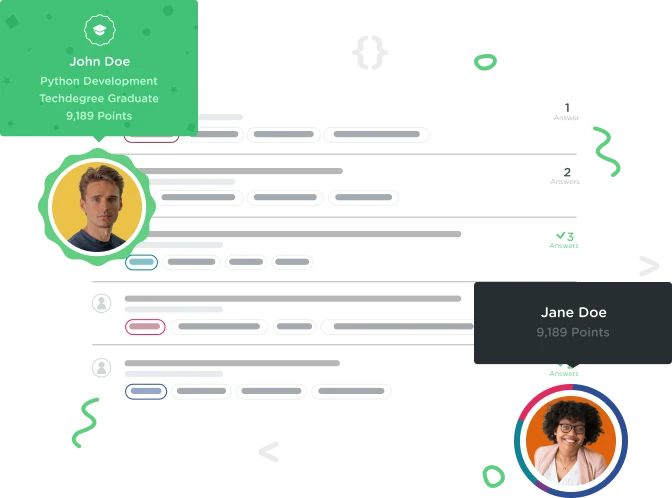
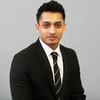
Mahmud Hussain
1,287 Pointsvalidating length- rspec
require 'spec_helper'
describe "Creating todo lists" do
it "redirects to the todo list index page on success" do
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with: "My todo list"
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content("My todo list")
end
it "Diplays an error when the todo list has a title less than 3 characters" do
expect(TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with: "Hi"
fill_in "Description", with: "This is what I'm doing today."
click_button "Create Todo list"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today")
end
it "displays an error when the todo list has no description" do
expect(TodoList.count).to eq(0)
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with: "Grocery list"
fill_in "Description", with: ""
click_button "Create Todo list"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery list")
end
end
when i do the command
bin/rspec spec/features/todo_lists/create_spec.rb
i get 1 failure
[deprecated] I18n.enforce_available_locales will default to true in the future. If you really want to skip validation of your locale you can set I18n.enforce_available_locales = false to avoid this message.
..F
Failures:
1) Creating todo lists displays an error when the todo list has no description
Failure/Error: expect(page).to have_content("error")
expected to find text "error" in "Todo list was successfully created. Title: Grocery list Description: Edit | Back"
# ./spec/features/todo_lists/create_spec.rb:46:in `block (2 levels) in <top (required)>'
Finished in 0.44264 seconds
3 examples, 1 failure
I dont understand why i get this error?
Thanks
3 Answers
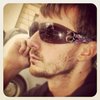
Adam Sackfield
Courses Plus Student 19,663 PointsThe error is telling you that "it expected the page to have the content 'error' but it found the text 'Todo list was successfully created. Title: Grocery list Description: Edit | Back' " instead. My guess is that you have not yet added the validation to the TodoList Modal.
validates :description, presence: true
The above code should enable the test to pass
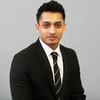
Mahmud Hussain
1,287 PointsOH WOW! thanks a lot Adam i found the mistake now! i had validate instead of validates! thanks a lot buddy! can finally move on :)
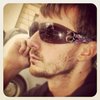
Adam Sackfield
Courses Plus Student 19,663 PointsGlad to help
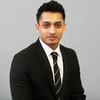
Mahmud Hussain
1,287 PointsHi Adam,
I have added that to my model, here it is:
class TodoList < ActiveRecord::Base
validates :title, presence: true
validates :title, length: {minimum: 3}
validate :description, presence: true
end
but i still get the error?