Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial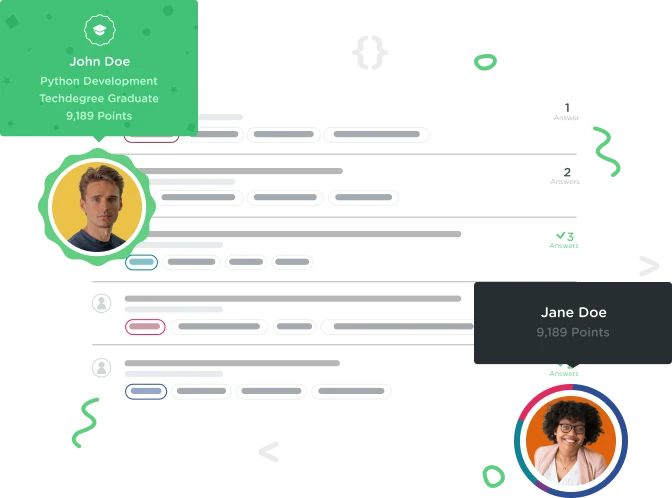

Dongyun Cho
2,256 PointsValueError!
from random import randint
rand_num = randint(1,9)
def try_game():
try_again= input("Game Over. Try Again? Yes or No: ")
if try_again.upper() =='Y' or try_again =="YES":
game(3)
elif try_again.upper() =='N' or try_again =="NO":
print("Ok ByeBye")
else:
print("what? You should type clearly!")
try_game()
def game(total):
global rand_num
print("SO LET'S START THE GAME.")
for i in range(total):
player_num = int(input("Guess the number of 1-9 : "))
if type(player_num) != int:
print("HeyHey what's going on? Enter a NUMBER between 1-9.")
#How can counting this 'except' be ignored?
elif player_num == rand_num:
print("YOU GoT IT! {}".format(rand_num))
break
else:
print("""
Sorry, You missed.
You have {} left times.
""".format(total-i-1))
try_game()
game(3)
this is my code and I got ValueEorror message!
for i in range(total):
player_num = int(input("Guess the number of 1-9 : "))
if type(player_num) != int:
print("HeyHey what's going on? Enter a NUMBER between 1-9.")
this line doesn't accept something not integer, at the FIRST loop. when I set string at the second or more loop. it runs fine .
what is the problem in my code? and please explain about it, thanks!
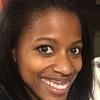
nicole lumpkin
Courses Plus Student 5,328 PointsYou might want to rethink this using a while loop. If you want to add the functionality that you only have a certain number of guesses you can set a variable to num_guesses and decrement that each time the loop where appropriate. So if your user enters something that's not an int, you wouldn't decrement the num_guesses variable in that branch of the code.
2 Answers
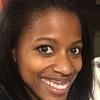
nicole lumpkin
Courses Plus Student 5,328 PointsHi Dongyun,
I'm actually on the same exercise as you and my approach to the problem was very different from yours. I'm not experienced enough to give you tips on how to debug your current code so I've simply posted my code. I hope you're able to draw inspiration from my code and make it your own :)
import random
def opening_greeting():
# -> print
print("Welcome." + "\n")
def get_valid_guess():
# -> int
while True:
try:
user_num = int(input("Guess a whole number between 1 and 10" + "\n" + "> "))
if 1 <= user_num <= 10:
break
else:
print("Invalid input. Your number must be between 1 and 10.")
continue
except ValueError:
print("Invalid input. It must be a number between 1 and 10.")
return user_num
def correct_guess(user_guess, secret_num):
# -> bool
if user_guess == secret_num:
return True
def play_again():
#-> bool
while True:
play_again = input("Would you like to play again? Enter Y or N." +\
"\n" + "> ")
if play_again not in ["Y", "N", "y", "n"]:
print("Invalid command. Please enter Y/N.")
else:
break
if play_again in ["Y", "y"]:
return True
else:
return False
def closing_goodbye():
print("--------")
print("Goodbye!")
print("--------")
def play_numberGame():
opening_greeting()
secret_num = random.randint(1,10)
num_guesses = 4
while num_guesses > 0:
user_guess = get_valid_guess()
if correct_guess(user_guess, secret_num):
print("You've guessed my number!!")
choice = play_again()
if choice:
play_numberGame()
else:
break
elif num_guesses == 1:
print("Sorry you are our of guesses.")
choice = play_again()
if choice:
play_numberGame()
break
elif user_guess > secret_num:
print("You guessed too high. You have {} guesses left. ".format(num_guesses - 1)\
+ "Guess again!")
num_guesses -= 1
else:
print("You guessed too low. You have {} guesses left. ".format(num_guesses - 1)\
+ "Guess again!")
num_guesses -= 1
closing_goodbye()
play_numberGame()

Dongyun Cho
2,256 PointsThank you all! Now I got concept about while and except stuffs! And Nicole, your code was so helpful!! Thanks again guys!
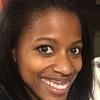
nicole lumpkin
Courses Plus Student 5,328 PointsYou're welcome ;)
Julio Garcia
1,944 PointsJulio Garcia
1,944 PointsYou need to do a exception block to test if is a integer number or other thing, Something like these
solution