Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial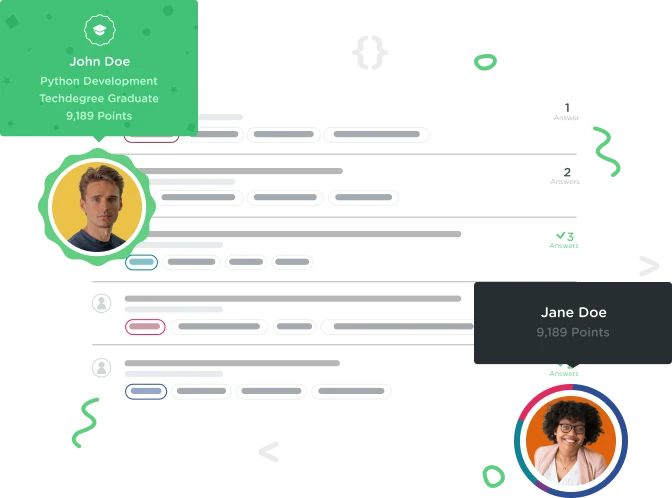

Ravindra Arya
79 PointsValueError in executing the code
This is the code I am trying to run:
import csv
input_file = "C:/Users/.../Google Drive/iris.csv"
with open(input_file, 'r') as iris_data:
irises = list(csv.reader(iris_data))
colors = {"Iris-setosa": "#2B5B84", "Iris-versicolor": "g", "Iris-virginica": "purple"}
from itertools import groupby
for species, group in groupby(irises, lambda i:i[4]):
categorized_irises = list(group)
sepal_lengths = [float(iris[0]) for iris in categorized_irises]
sepal_widths = [float(iris[1]) for iris in categorized_irises]
plt.scatter(sepal_lengths,sepal_widths,s=10,c=colors[species],label=species)
plt.title("Iris Data Set", fontsize=12)
plt.xlabel("sepal length (cm)",fontsize=10)
plt.ylabel("sepal width (cm)",fontsize=10)
plt.legend(loc="upper right")
plt.show()
And, I am getting this error:
Traceback (most recent call last):
File "<ipython-input-21-25a00ab199e6>", line 4, in <module>
sepal_lengths = [float(iris[0]) for iris in categorized_irises]
ValueError: could not convert string to float: sepal length
I will appreciate if someone can tell me the error in my code. Thanks for your consideration.
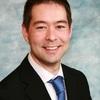
Mark Chesney
11,747 PointsHi Ravindra, I'm seeing the same problem as you, even with the answer below taken into account, so I've begun a new thread. I feel your pain, my friend!
1 Answer
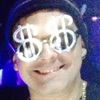
Mustafa Başaran
28,046 PointsHello Ravindra,
Your code is perfect but please note that the last row of iris.csv file is blank. That is why Ken removed it before he started the loop with groupby method. So, please add
irises.pop()
before the loop and after you read the file into a list of lists with csv module. Then, it should work OK. I hope this helps.
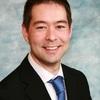
Mark Chesney
11,747 PointsUnfortunately, I had the irises.pop()
line and I still see the error. :( Please let me know if you have any further ideas, by any chance?
Timothy Long
Front End Web Development Techdegree Student 6,833 PointsTimothy Long
Front End Web Development Techdegree Student 6,833 PointsI've never used the CSV module in Python and am not familiar with the projects on treehouse. However it looks like Python is trying to covert the string
sepal length
to a float when your code runs:sepal_lengths = [float(iris[0]) for iris in categorized_irises]
When you call
iris[0]
it appears that index in the list is a string and you cannot convert a string of text into anint
or afloat
.I hope this is at least somewhat helpful. If you'd like to give me more context I can try to help. You may simply be calling the wrong index. To check you can try printing
categorized_irises
to see it's contents and ensure you're pulling the correct index. But again as a disclaimer I'm not familiar with the treehouse courses in Python. I'm only familiar with Python. (2 & 3)