Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial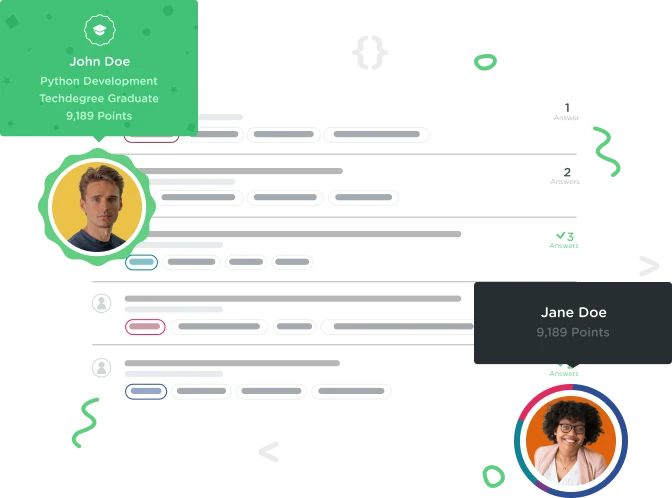

Minseok Kim
2,708 PointsValueError not caught..?
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should i add {}?\n"
"press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print ("-"*10)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()
I added orange, apple, peach, and then when i added grape with position "a", it just added grape to the end of the list.
1 Answer
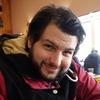
Eric M
11,546 PointsHi Minseok,
A ValueError is raised, but you've written an except
block to handle it.
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
Your try
block raises a ValueError because you can't cast strings to int in this manner, then your except block says to set position
to None
rather than terminate the program. As position
is None
, the conditional branch is false and we move to the else
clause's branch, which runs the append
method on shopping_list
.
I'd like to point out here you're passing the append
method new_item
which is in the global scope, it's part of your while
loop. It would be more consistent to instead pass item
the parameter of add_to_list
that you use elsewhere in this function. If add_to_list
was called by another function instead of a while loop with global scope, this reference to new_item
wouldn't resolve.
If you want to see something to be sure the ValueError is executing, you could make some changes to `add_to_list()
like so:
def add_to_list(item):
show_list()
err = ""
if len(shopping_list):
position = input("Where should i add {}?\n"
"press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
err = " - added after ValueError"
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item + err)
show_list()
In your example this would add grape to the end of the list as grape - added after ValueError
. Not very good to show to a user, but potential helpful during testing and debugging!
Cheers,
Eric