Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial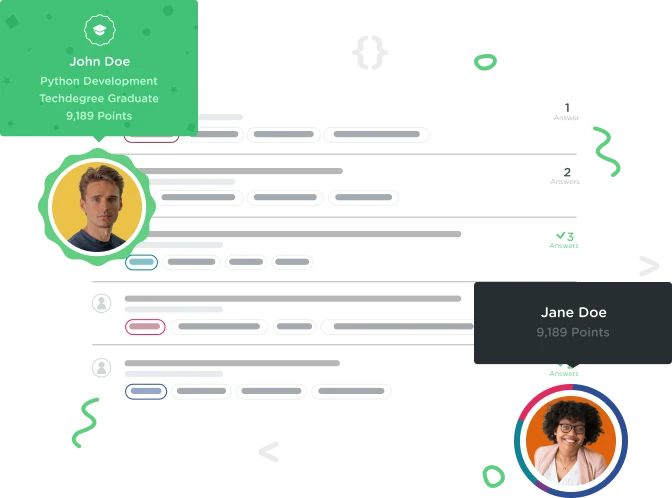

Li Mbo
5,096 Pointsvar and let
In this case: func sayHello(personName: String) -> String { let greeting = "Hello, " + personName + "!" return greeting }
Why do we use let instead of var for the variable greeting? The variable greeting should generate different strings depending on different inputs, so why do we use let instead of var? and how can a constant change its value after giving it the first input?
Source: Apple Documentations https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Functions.html#//apple_ref/doc/uid/TP40014097-CH10-ID158
2 Answers

martin2s
1,937 PointsHi amir, I'm still learning swift myself, so please take my answer with a pinch of salt, as i may be wrong.
Firstly, from what i understand, let is preferred over var, because using a constant allows the compiler to optimise code and memory allocation.
But I think you're also asking why Let is being used in this specific example, as the function will clearly be called more than once. So in effect, you're asking - how can we call the function more than once with a Let value that appears to be fixed forever as a constant??!! The reason for this is that the constant "greeting" is local to the function - ie "greeting" is not recognised as a variable outside of the function. Greeting only exists within the function. The return value from the call is recognised and received - but you will have to assign this to a variable explicitly (see my example below).
Thus, because the constant Greeting is local to the function, it is only ever assigned once, so a let assignment is perfectly acceptable. Within the scope of the sayHello function, its value never changes. The next time you call the function, you are creating a completely new instance of the variable, so again, a new let assignment makes perfect sense (but the new instance of greeting has no relation to the old instance). Within the scope of the sayHello function, the constant never changes its value (and once the function returns its value, the memory allocated to that instance is deleted entirely, presumably). ie every time you call the function, you're creating a completely new instance of the variable greeting.
To backup my thinking.... If you add the following line to your code (outside of your function definition!!), then you will get an error: println("Greeting is: (greeting)") Error - use of unresolved identifier greeting. This proves that greeting does not exist as a variable outside of the function.
In contrast, if i now instead define a new variable outside of the function definition, then it works without an error. func sayHello(personName: String) -> String { let greeting = "Hello, " + personName + "!" return greeting }
var greeting2 = sayHello("Tom") println("this is the greeting (greeting2)")
var greeting3 = sayHello("Amir") println("this is the greeting (greeting3)")
hope this helps,
cheers, martin.

Myles Linder
2,040 PointsHey Amir,
The greeting constant evaluates to a different value each time you run the function because Xcode first evaluates the the argument, personName, and then evaluates the expression for greeting.
The fact that it is a variable or a constant only comes in to play if you intended to change it's literal value, which is: "Hello, " + personName + "!"

Li Mbo
5,096 PointsThis was helpful, thank you Myles. However, what difference would it make if we use var rather than let? is it better for the memory allocation or something related to that?
Li Mbo
5,096 PointsLi Mbo
5,096 PointsThis helped a lot, thank you so much Maritn!