Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial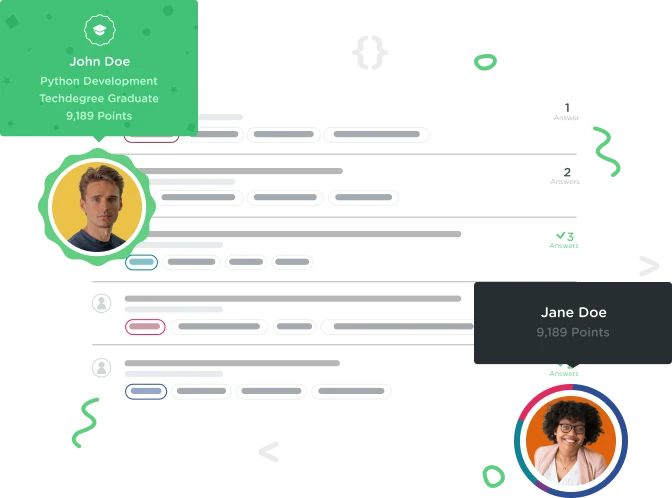

Kyle Southerland
UX Design Techdegree Student 11,117 PointsVar and Let in for loops
I understand scope, but perhaps I don't understand what goes on under the hood with for loops. The following code is from the JavaScript tutorial on Const and Let. Using "var" all buttons will output "Button 10 Pressed" when clicked. Using "let" they will correctly display "Button (insert number of button) Pressed." I was under the impression that once the event listener was added during each iteration that it was pretty much set in stone. Based on the output though, it's almost like nothing is really saved until the loop has completed. I'm not seeing how i being in global scope really affects the value in the event listener after it has been processed through the loop and has moved on to the next iteration.
<h1>Buttons</h1> <button>Button 0</button> <button>Button 1</button> <button>Button 2</button> <button>Button 3</button> <button>Button 4</button> <button>Button 5</button> <button>Button 6</button> <button>Button 7</button> <button>Button 8</button> <button>Button 9</button> <button>Button 10</button>
<script>
const buttons = document.getElementsByTagName("button");
for (var i = 0; i < buttons.length; i++) {
const button = buttons[i];
button.addEventListener("click", function() {
alert("Button " + i + " Pressed");
});
}
</script>
2 Answers
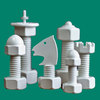
Steven Parker
229,783 PointsThe scope makes all the difference.
The variable "i" in the event handler is not evaluated when the handler is established, but later when the event occurs. So if the variable has global scope, it will now have a different value that it did when the handler was set up. As you can see from running this example, it will have the value left over from finishing the loop.
On the other hand, if you use let to declare the loop index, the scope will be limited to inside the loop, and the value will be the same when the event occurs as when the handler was set up.
Make sense now?

Kyle Southerland
UX Design Techdegree Student 11,117 PointsAlexander Davison thanks for the heads up, new to the community.
Kyle Southerland
UX Design Techdegree Student 11,117 PointsKyle Southerland
UX Design Techdegree Student 11,117 PointsIt does, thanks!
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsPlease provide a Best answer for Steven Parker. Thanks! ~Alex