Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial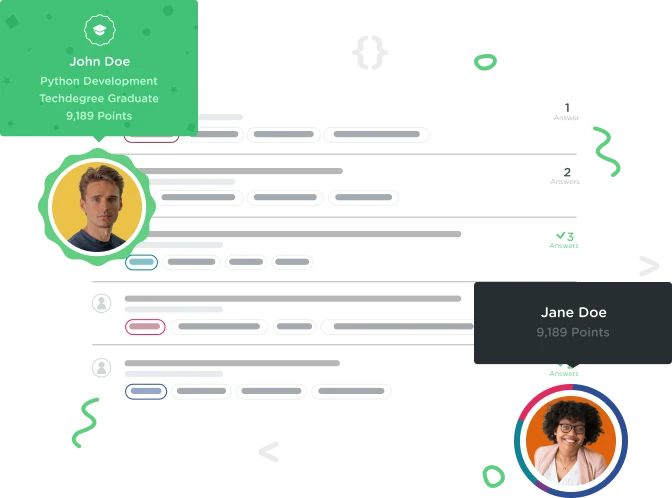
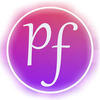
Andrew Bergsma
880 Pointsvar europeanCapitals: [String] = [] var asianCapitals: [String] = [] var otherCapitals: [String] = [] ?
Please for the love of Earth tell me what is wrong with my code below, thank you for your time:
var europeanCapitals: [String] = [] var asianCapitals: [String] = [] var otherCapitals: [String] = []
let world = [ "BEL": "Brussels", "LIE": "Vaduz", "BGR": "Sofia", "USA": "Washington D.C.", "MEX": "Mexico City", "BRA": "Brasilia", "IND": "New Delhi", "VNM": "Hanoi"]
Let countryCodes = ["BEL", "LIE", "BGR", "USA", "MEX", "BRA", "IND", "VNM"]
for countryCode in world { // Enter your code below switch countryCode { case "BEL", "LIE", "BGR": europeanCapitals.append("Brussels", "Vaduz", "Sofia") case "USA", "MEX", "BRA": otherCapitals.append("Washington D.C.", "Mexico City", "Brasilia") case "IND", "VNM": asianCapitals.append("New Delhi", "Hanoi") default: otherCapitals("country code does not exist") } // End code }
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
Let countryCodes = ["BEL", "LIE", "BGR", "USA", "MEX", "BRA", "IND", "VNM"]
for countryCode in world {
// Enter your code below
switch countryCode {
case "BEL", "LIE", "BGR": europeanCapitals.append("Brussels", "Vaduz", "Sofia")
case "USA", "MEX", "BRA": otherCapitals.append("Washington D.C.", "Mexico City", "Brasilia")
case "IND", "VNM": asianCapitals.append("New Delhi", "Hanoi")
default: otherCapitals("country code does not exist")
}
// End code
}
2 Answers

Shade Wilson
9,002 PointsThe switch statement is actually simpler than what you're trying to do. You don't have to create another variable called countryCodes
because you can iterate through the dictionary by its key-value pairs. These are innate properties of dictionaries, so you don't need to create them yourself.
You should use what the challenge gives you by default:
for (key, value) in world {
// Enter your code below
// End code
}
Here the for loop goes through every key-value pair in world
. You could do it by declaring an array that has all the keys of the dictionary the way you're trying to do it, but there's no need to complicate things if we don't have to.
As Jack said, the main problem with your code is that if "BEL"
is matched, all three of the european capitals would be appended to the array. By the end of the for loop, europeanCapitals
would have three duplicates of each capital, which we don't want. The same is true for the other two cases. Instead, all you have to do is append value
like so:
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
Also, your default statement is wrong. I think it makes the most sense to take out the case of other capitals and instead append to otherCapitals
as the default case because any capital that's not European or Asian would fall into the other category. It's ok to have a specific case for otherCapitals
, but like Jack said you should have a print statement in the defalut saying "country code does not exist". Trying to put that string inside otherCapitals
will throw an error.
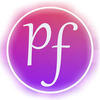
Andrew Bergsma
880 PointsI have since removed my multi value syntax for my "BEL" case but I'm now getting an error back on ALL cases "Expression pattern of type 'String' cannot match values of type '(key: String, value: String)'"
Revised code below:
```var europeanCapitals: [String] = [] var asianCapitals: [String] = [] var otherCapitals: [String] = []
let world = [ "BEL": "Brussels", "LIE": "Vaduz", "BGR": "Sofia", "USA": "Washington D.C.", "MEX": "Mexico City", "BRA": "Brasilia", "IND": "New Delhi", "VNM": "Hanoi"]
for BEL in world { // Enter your code below switch BEL { case "BEL", "LIE", "BGR": europeanCapitals.append("Brussels", "Vaduz", "Sofia") case "USA", "MEX", "BRA": otherCapitals.append("Washington D.C.", "Mexico City", "Brasilia") case "IND", "VNM": asianCapitals.append("New Delhi", "Hanoi") default: print("country code does not exist") } }```

Shade Wilson
9,002 PointsYou need to use a tuple to loop through world
, or else you won't be able to pattern match and will run into the error you're seeing:
for (key, value) in world {
// switch statement here
}
You can read more about tuples here in the Apple documentation
You need to do this because you're matching on the key (the 3-letter country code), but you need to append the value (the city name as a string). Also, you should be appending just the value as I showed above. You shouldn't manually put in the values for the city names.
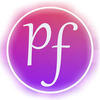
Andrew Bergsma
880 PointsOk, I figured out. I made it more complex than it needed to be! LOL
Without trying to give it away.. The issue was my for in loop.
for (key, value) in world { switch key {
The rest of the code was basic. The moderators greatly helped me at least get to the point of being able to understand what I'm actually trying to get the code to do. From there I was able to go back to the instructions and work my way down. Took me awhile but with the moderators help I was able to persevere, thank you!! Happy coding!(well sometimes lol)
Jack Baer
5,049 PointsJack Baer
5,049 PointsYour problem is in the switch statement. Say countryCode was set to "BEL". The way you have it set up is to where Brussels, Vaduz, and Sofia get appended. Not just Brussels. Also, the default is strange. Maybe just have it do something that doesn't affect anything, such as print a message.