Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial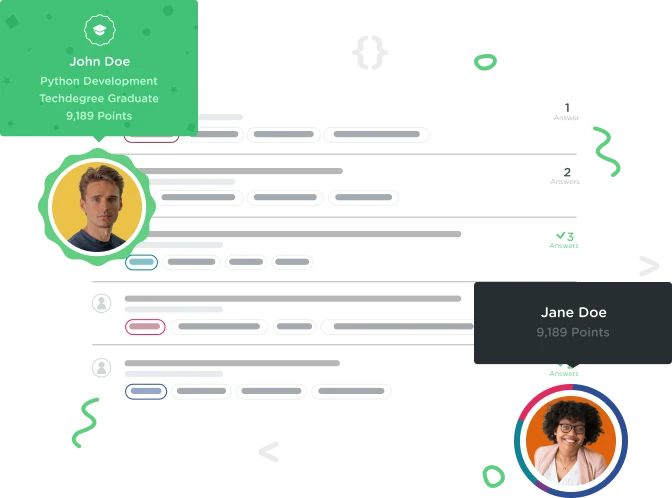

orange sky
Front End Web Development Techdegree Student 4,945 Pointsvar_dump();
Hello,
A regular var_dump
$bool = TRUE;
var_dump($bool);
$bool = FALSE;
var_dump($bool);
A typecast version
$bool = TRUE;
$bool = FALSE;
var_dump((bool) "abc");
It seems to me that var_dump has two functions. The first one is to check whether a variable is true or false. And the second function is to typecast. I am not sure of the meaning of typecast/
Can you please explain the difference between the first code(a regurlar var_dumpt) and the second code (A typecase version)?
2) In the typecast version, I don't understand how $bool stores the FALSE value, but the var dump evalulates to true.
Thanks
3 Answers
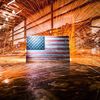
Andrew Shook
31,709 PointsVar_dump is simply a function that will dump information about a variable. So if you var_dump a variable holding an object, all the methods and properties about the object will be printed to the screen. It's not just for boolean values. You can see more examples with var_dump on the PHP Manual site
Now typecasting is simply a way for you to tell php what variable type you want a variable treated as. PHP is a loosely typed language, mean you don't have to tell php what kind of variable you are declaring when you declare a variable. Instead, you put a "$" and php will figure out whether the variable is a sting, integer, float, array, object, or whatever. By typecasting, you are telling php, "I don't care what you think it is, treat this variable as..." whatever you typecasted it to. In the example you gave above:
<?php
$bool = TRUE;
var_dump($bool);
$bool = FALSE;
var_dump((bool) "abc");
?>
The var_dump will return "true", because the string is not empty. Also, the "(bool)" you are putting in front of the string "abc" is not the variable $bool, but is just the way you typecast a variable to be a boolean.

Bogdan Filippov
1,019 PointsGod bless you! Thanks for explanation!

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Andrew!
Thank you so much! I totally got.
As for the funky looking typecasting, I think I have seen it in Java, but anyway, I now understand var dump().
Cheers!!

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Andrew,
This is strange but your message did not come to my hotmail account; I found out you replied because I was pressing a button in the classroom to read what other questions were posted. Ok, I wil check my setting...
As for var_dump, I see it is not just for boolean values, but I am just a little confused about typecasting. If I understand you:
1)This below is not typecasting, and the $bool is a variable that is holding a boolean value
var_dump($bool);
2) This is typecasting. The bool is not a variable but it setting "abc" to true like a bolean value???
var_dump((bool) "abc");
I thought a typecast was in this format: $num int = 3; $bool boolean = TRUE;
A typecast like this makes sense: $bool boolean = TRUE; but a typecast like this below is hard to understand that it is setting "abc" to true: var_dump((bool) "abc");
Cheers!!!
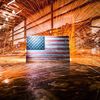
Andrew Shook
31,709 PointsYes, you are not typecasting in that instance.
The "(bool)" is not automatically setting the string to true. It simply tells PHP to evaluate the string and determine it's boolean value. In PHP, any non empty string is considered true. So that is why the string is converted to true when you typecast it.
I don't think I have ever seen typecasting in any language using that format, but that is definitely not how it is done in PHP.
Bogdan Filippov
1,019 PointsBogdan Filippov
1,019 PointsThanks for question, I had the same issue!