Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial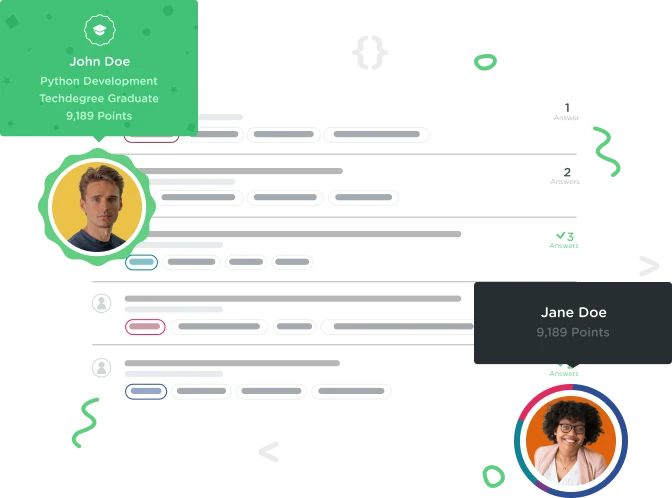

Sebastiaan van Vugt
Python Development Techdegree Graduate 13,554 PointsVariable in a loop is updated continuously - before it is supposed to do so.
Summarised my problematic code is sth like:
while True:
if aa:
if bb:
elif x:
elif y:
elif z:
else:
for a in range (0, (len(secret_word))):
for guess in guess_list:
if secret_list[a] == guess:
progress_list[a] = guess
if progress_list != progress_list2:
print("There is a '{}' in the secret word indeed :)\n".format(guess))
b +=1
else:
color.write("The letter '{}' is not in the secret word.\n".format(guess),"COMMENT")
progress_list2 = progress_list ```
The first time python goes through this loop it is possible that progress_list != progress_list2 but the second time this becomes impossible. The progress_list should be updated before progress_list2 and therefore it should be possible to continue entering if progress_list != progress_list2. When printing the progress_list and progress_list2 during the loop one can, however, see that only the first time around the lists can be different and that the second and following times they are updated simultaneously. Does anyone have an explanation for this?
The full code can be found here: https://codeshare.io/a3vPdg
Thanks a lot!
1 Answer

Sebastiaan van Vugt
Python Development Techdegree Graduate 13,554 PointsThis website helped me understand how variables are bound in Python: python-variables
These examples explain how variables are bound in Python:
Appending a will append b
a = [1, 2]
b = a
a.append(3)
print("a = {}".format(a))
print("b = {}".format(b))
result: a = [1, 2, 3] b = [1, 2, 3]
Variables are bound and not equated i.e. this works in one direction
a = [1, 2]
a = b
a.append(3)
print("a = {}".format(a))
print("b = {}".format(b))
result: NameError: name 'b' is not defined
a is bound anew
b = [9]
a = [1, 2]
a = b
a.append(3)
print("a = {}".format(a))
print("b = {}".format(b))
result: a = [9, 3] b = [9, 3]
a substitution in a will substitute in b
a = [1, 2]
b = a
a.append(3)
a[1]=9
print("a = {}".format(a))
print("b = {}".format(b))
result: a = [1, 9, 3] b = [1, 9, 3]
b is bound anew
a = [1, 2]
b = a
a.append(3)
a[1]=9
b = [10]
print("a = {}".format(a))
print("b = {}".format(b))
result: a = [1, 9, 3] b = [10]