Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial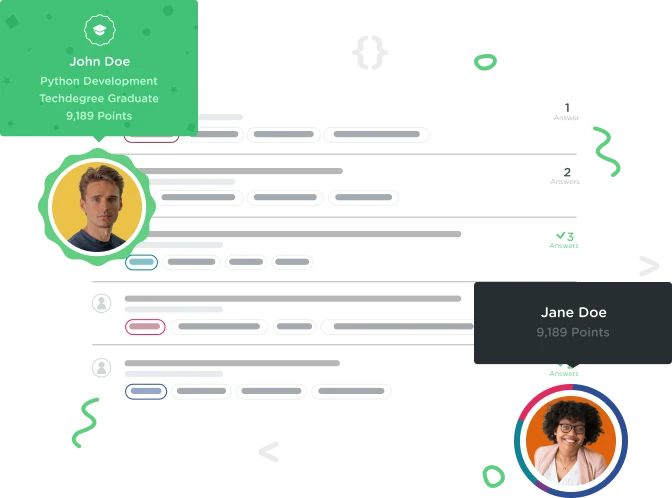
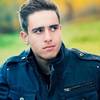
Unsubscribed User
14,547 PointsVariable in array doesn't seem to be updating
I'm refactoring my quiz code for the "Build a Quiz Challenge, Part 1" to use arrays.
Everything seems to work fine except the quizCongrats array. It displays on screen at the end of the quiz, but the quizScore variable is always 0. The quizTabulate function works fine and displays the right message depending on quiz score, so quizScore is updating correctly..
It's probably a simple spelling error or mis-remembering of how arrays work that's causing it, so any help I would appreciate. Thanks!
function print(message) {
document.write(message);
}
// Set user score to 0
var quizScore = 0;
// Questions
var quizQuestion = [
['How do you go up a folder level in Bash?', '..'],
['What would you type to output an error stream to grep in Bash using an anonymous pipe? Include all text from the stream selector command onwards. Search for "Error" and make grep ignore case', '2> >(grep -i error)'],
['What would you type to run the previously entered command as sudo?', 'sudo !!'],
['How do you resume a task stopped with CTRL-Z?', 'fg'],
['What is the MAC address of our router? (Hint: "arp -a" in cmd. You can use "ipconfig" to find the default gateway (router)', '50-6a-03-a7-05-35']];
// Score
var quizCongrats = [
'<h1>Congratulations you got ' + quizScore + ' out of 5 correct. You get the Gold Medal</h1>',
'<h1>Congratulations you got ' + quizScore + ' out of 5 correct. You get the Silver Medal</h1>',
'<h1>You got ' + quizScore + ' out of 5 correct. You get the Bronze Medal. Try harder next time.</h1>',
'<h1>You got ' + quizScore + ' out of 5 correct. You fail!</h1>'];
// Ask Questions
function quiz(input) {
for ( i = 0; i < quizQuestion.length; i += 1 ) {
if ( prompt(quizQuestion[i][0]) === quizQuestion[i][1] ) {
quizScore += 1;
}
}
return quizScore;
}
// Calculate Score
function quizTabulate(input) {
if ( quizScore === 5 ) {
return quizCongrats[0];
} else if ( quizScore > 2 ) {
return quizCongrats[1];
} else if ( quizScore > 0 ) {
return quizCongrats [2];
} else {
return quizCongrats[3];
}
}
print(quizTabulate(quiz()));
1 Answer

Daniel Samer
14,473 PointsYou Problem here is , that you declare those Arrays before the quizScore gets changed.
You have to declare this Array when the Quizscore is is already at its set Value.
function print(message) {
document.write(message);
}
// Set user score to 0
var quizScore = 0;
var quizCongrats = []
// Questions
var quizQuestion = [
['How do you go up a folder level in Bash?', '..'],
['What would you type to output an error stream to grep in Bash using an anonymous pipe? Include all text from the stream selector command onwards. Search for "Error" and make grep ignore case', '2> >(grep -i error)'],
['What would you type to run the previously entered command as sudo?', 'sudo !!'],
['How do you resume a task stopped with CTRL-Z?', 'fg'],
['What is the MAC address of our router? (Hint: "arp -a" in cmd. You can use "ipconfig" to find the default gateway (router)', '50-6a-03-a7-05-35']
];
function declareCongratz() {
quizCongrats = [
'<h1>Congratulations you got ' + quizScore + ' out of 5 correct. You get the Gold Medal</h1>',
'<h1>Congratulations you got ' + quizScore + ' out of 5 correct. You get the Silver Medal</h1>',
'<h1>You got ' + quizScore + ' out of 5 correct. You get the Bronze Medal. Try harder next time.</h1>',
'<h1>You got ' + quizScore + ' out of 5 correct. You fail!</h1>'];
}
// Ask Questions
function quiz(input) {
for (i = 0; i < quizQuestion.length; i += 1) {
if (prompt(quizQuestion[i][0]) === quizQuestion[i][1]) {
quizScore += 1;
}
}
return quizScore;
}
// Calculate Score
function quizTabulate(input) {
declareCongratz()
if (quizScore === 5) {
return quizCongrats[0];
} else if (quizScore > 2) {
return quizCongrats[1];
} else if (quizScore > 0) {
return quizCongrats[2];
} else {
return quizCongrats[3];
}
}
print(quizTabulate(quiz()));
This is the Solution that is the nearest to your Code.
That is caused by the declaration of the Array. An Array Item can only hold one type of an Object so when you merge that String with the String in the Variable you get a new (static String) with both Strings concluded. The Array Object doesnt know afterwards that it was built from a string and a variable, its just a string.
Unsubscribed User
14,547 PointsUnsubscribed User
14,547 PointsThanks a lot. I'm learning about Arrays right now so I still need to memorize the specifics. That makes total sense.