Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial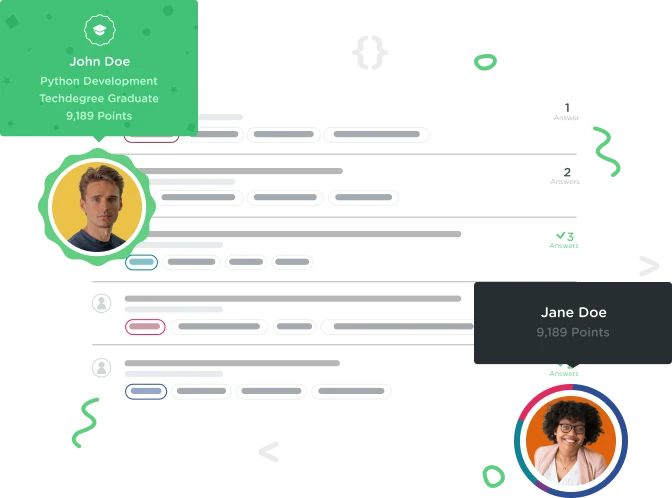

gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 Pointsvariable returned stays unusable
Hi, I am trying to create a helper function for an event listener. In my helper function, I am returning a value that's required by my event listener. Unfortunately, all I get is a "Uncaught ReferenceError: nameIsValid is not defined" type error.
Below is my code, I would appreciate any help :)
function nameVerifier(nameForm) {
const nameValue = nameForm.value;
const nameIsValid = /^[a-zA-Z]+ ?[a-zA-Z]*? ?[a-zA-Z]*?$/.test(nameValue);
console.log('name: ' + nameIsValid);
return nameIsValid
}
form.addEventListener('submit', (e) => {
e.preventDefault();
nameVerifier(nameForm);
if (nameIsValid != true) {
e.preventDefault();
}
});
3 Answers
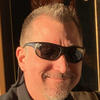
Peter Vann
36,425 PointsHi Gael!
I think I see the problem(s).
It does NOT appear that you are storing the return value from your function.
nameForm.value won't work, either...
function nameVerifier(nameForm) {
const nameValue = nameForm.value; // This is wrong
const nameIsValid = /^[a-zA-Z]+ ?[a-zA-Z]*? ?[a-zA-Z]*?$/.test(nameValue);
console.log('name: ' + nameIsValid);
return nameIsValid
}
form.addEventListener('submit', (e) => {
e.preventDefault();
nameVerifier(nameForm); // Not storing the return value
if (nameIsValid != true) {
e.preventDefault();
}
});
Try something like this:
function nameVerifier(nameForm) {
console.log(nameForm.value); // undefined
console.log(nameForm.getElementsByTagName('INPUT')[0].value );
const nameValue = nameForm.getElementsByTagName('INPUT')[0].value; // Not nameForm.value
console.log(nameValue);
const nameIsValid = /^[a-zA-Z]+ ?[a-zA-Z]*? ?[a-zA-Z]*?$/.test(nameValue);
console.log('name: ' + nameIsValid);
return nameIsValid
}
form.addEventListener('submit', (e) => {
e.preventDefault();
// store nameVerifier's return value in a variable
let name_Is_Valid = nameVerifier(e.target); // You need to pass in e.target to this function
if (name_Is_Valid != true) {
console.log("Not Valid!");
e.preventDefault();
} else {
console.log("Valid!");
e.preventDefault();
}
});
I altered some of the code for clarity, fixed other issues, and got it to work...
(Keep in mind: e.target is the form itself.)
You can test it here:
https://www.w3schools.com/js/tryit.asp?filename=tryjs_array
Copy this code:
<!DOCTYPE html>
<html>
<body>
<form id="form">
<label>Test field: <input type="text"></label>
<br><br>
<button type="submit">Submit form</button>
</form>
<p id="log"></p>
<script>
function nameVerifier(nameForm) {
console.log(nameForm.value); // undefined
console.log(nameForm.getElementsByTagName('INPUT')[0].value );
const nameValue = nameForm.getElementsByTagName('INPUT')[0].value;
console.log(nameValue);
const nameIsValid = /^[a-zA-Z]+ ?[a-zA-Z]*? ?[a-zA-Z]*?$/.test(nameValue);
console.log('name: ' + nameIsValid);
return nameIsValid
}
form.addEventListener('submit', (e) => {
e.preventDefault();
// store nameVerifier's return value in a variable
let name_Is_Valid = nameVerifier(e.target); // You need to pass in e.target to this function
if (name_Is_Valid != true) {
console.log("Not Valid!");
e.preventDefault();
} else {
console.log("Valid!");
e.preventDefault();
}
});
</script>
</body>
</html>
Paste it into the left pane and run it - check the console on the right pane (right-click, inspect, console tab)
I hope that helps.
Stay safe and happy coding!
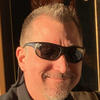
Peter Vann
36,425 PointsNo worries, Gael - happy to help!
BTW, I tried it with Betty and got Valid!
I tried 123 and got Not Valid!
I hope that helps.
Stay safe and happy coding!

gael blanchemain
Full Stack JavaScript Techdegree Student 8,843 PointsHey Peter, your answer is more than what I could hope for, thank you! Will try it asap.