Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial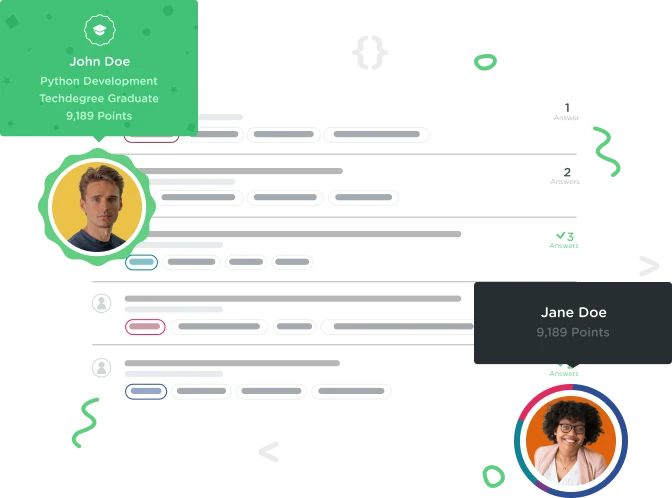

Jordan Eliastam
2,178 PointsVariable scope
I am confused by the scope of variables in this project. It seems like monster, player and door are initialized at a global scope, yet we are passing player as an argument to the functions. Also, once we pass it, it has the same name in the called function as the global variable. Please elaborate! Thank you!
2 Answers

Lewis Cowles
74,902 PointsIn a real world OOP application the player would be an object with properties, methods etc, being passed into each level by the game, but in this example it is a tuple with two numbers indicated to be X and Y; so I think we have to be a little forgiving.
As for name within functions, I think this might be confusing you because internally to the functions the same name was chosen, I think to help you track what was going on with the player... Essentially the functions have no clue about the global player (or at least they wouldn't in any real world app), so if you rename player to pos within each of the functions or remove it, you will of course get a better idea of what the functions are doing with a position of a player that they are passed.
I Suppose this is not exactly helped by referencing other objects from the global namespace either, but I think the example was solid for it's intended purpose (playing with tuples as opposed to application or game design). I modified the example so it used all global vars for you as you can see it still works, but unlike Kenneth's version because moving the player does not take player via arguments creating a real-world app would be much harder ;).
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(CELLS)
door = random.choice(CELLS)
start = random.choice(CELLS)
if monster == door or monster == start or door == start:
return get_locations()
return monster, door, start
def move_player(move):
# pos = (x, y)
x, y = player
if move == 'LEFT':
y -= 1
elif move == 'RIGHT':
y += 1
elif move == 'UP':
x -= 1
elif move == 'DOWN':
x += 1
return x, y
def get_moves():
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
# pos = (x, y)
if player[1] == 0:
moves.remove('LEFT')
if player[1] == 2:
moves.remove('RIGHT')
if player[0] == 0:
moves.remove('UP')
if player[0] == 2:
moves.remove('DOWN')
return moves
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(CELLS):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player:
print(tile.format('X'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player:
print(tile.format('X|'))
else:
print(tile.format('_|'))
monster, door, player = get_locations()
print("Welcome to the dungeon!")
while True:
moves = get_moves()
print("You're currently in room {}".format(player))
draw_map()
print("You can move {}".format(moves))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in moves:
player = move_player(move)
else:
print("** Walls are hard, stop walking into them! **")
continue
if player == door:
print("You escaped!")
break
elif player == monster:
print("You were eaten by the grue!")
break

Jordan Eliastam
2,178 PointsYea, that is what I suspected. Thank for your your help, I appreciate it!
Lewis Cowles
74,902 PointsLewis Cowles
74,902 PointsThanks for selecting mine as the Best Answer, I tried to make it comprehensive, Happy New Year!
Jordan Eliastam
2,178 PointsJordan Eliastam
2,178 Pointsno problem, it was the only one! ha, but it did clear up my confusion. Thank you and happy new year to you as well!