Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial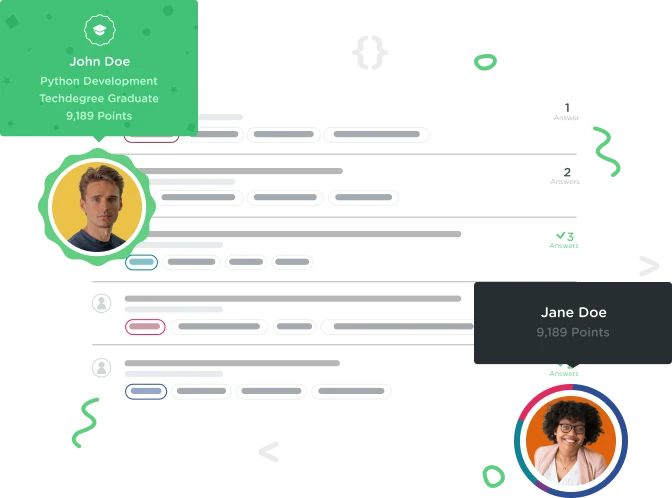

Chris Feltus
3,970 PointsVariable Scope
I was under the impression that if you have a global variable, and create another variable locally with the same name within a function, the two can coincide because they are within different scopes.
This doesn't seem to be the case since the below code does not work. Notice I define var $email globally and use $email again within the function.
var $fname = $('#fname');
var $lname = $('#lname');
var $email = $('#email');
$('.btn-submit').click(function(event){
event.preventDefault();
var $fval = $fname.val();
var $lval = $lname.val();
var $email = $email.val();
if( $fval == ''){
alert( 'please fill out the first name' );
}
if( $lval == ''){
alert( 'please fill out the last name' );
}
if( $email == ''){
alert( 'please fill out the last name' );
}
if( $fval && $lval && $email ){
alert( 'Thank you! Your cat has been sent!' )
}
});
But when I change the variable name from $email to $eval within the function, the code runs just fine as shown in the code below.
var $fname = $('#fname');
var $lname = $('#lname');
var $email = $('#email');
$('.btn-submit').click(function(event){
event.preventDefault();
var $fval = $fname.val();
var $lval = $lname.val();
var $eval = $email.val();
if( $fval == ''){
alert( 'please fill out the first name' );
}
if( $lval == ''){
alert( 'please fill out the last name' );
}
if( $eval == ''){
alert( 'please fill out the last name' );
}
if( $fval && $lval && $eval ){
alert( 'Thank you! Your cat has been sent!' )
}
});
Thanks for the clarification.
2 Answers

Damien Watson
27,419 PointsHi Chris,
You can have a global and local but it may be because you have redefined it, the reference to $email may be taking over.
var $abc = 10;
function doSomething() {
var $abc = $abc +5; // --> NAN = NAN + 5;
}
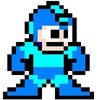
Robert Richey
Courses Plus Student 16,352 PointsHi Chris,
I believe what you are experiencing is known as variable hoisting. Here is the relevant quote from the link:
Because variable declarations (and declarations in general) are processed before any code is executed, declaring a variable anywhere in the code is equivalent to declaring it at the top. This also means that a variable can appear to be used before it's declared. This behavior is called "hoisting", as it appears that the variable declaration is moved to the top of the function or global code.
When a variable is declared and assigned to in the same line, there are really two steps going on.
function foo() {
var $email = $email.val();
}
// becomes
function foo() {
var $email; // $email is declared and is undefined
$email = $email.val();
}