Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial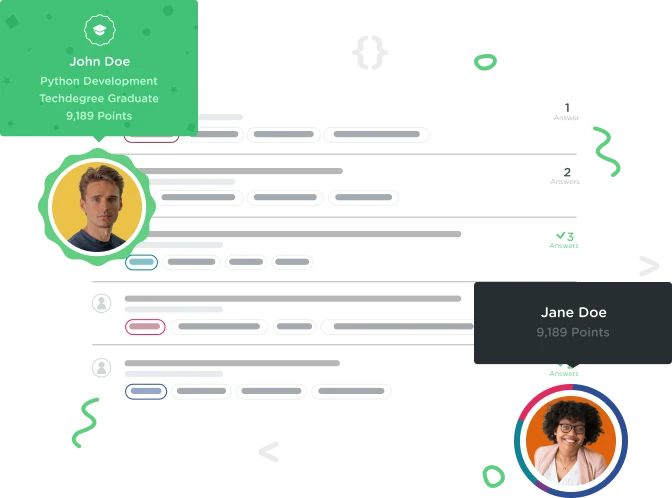

Winston Kaseke
874 PointsVariable Scope Where am i getting it wrong
Compiling the below code currently produces the error: "The name 'output' does not exist in the current context". This error is the result of improper variable scoping. The output variable is declared twice: once within the if statement's curly braces and again within the else statement's curly braces. Remember, variables in C# can only be used within the curly braces that they are declared within. The last line of code in this program, Console.WriteLine(output);, is attempting to use a variable named output, which doesn't exist outside of the if/else statement's curly braces. To fix this error: Declare the output variable just before the if statement and assign it to an empty string (i.e. ""). Remove the string data types from the output variables within the if/else statement's curly braces
My Answer using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
if (input == "quit")
{
output = "Goodbye.";
}
string output = Console.ReadLine();
if
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
if (input == "quit")
{
output = "Goodbye.";
}
string output = Console.ReadLine();
if
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}
1 Answer
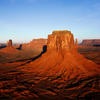
Tim Strand
22,458 PointsYou declared output midway down in the program. Just declare it as a string at the top of the program and get rid of the declaration inbetween the 2 ifs. then make them an if else statement instead.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
string output = "";
if (input == "quit")
{
output = "Goodbye.";
}
else
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
}
}
Winston Kaseke
874 PointsWinston Kaseke
874 PointsThe following error will pop up when viewing: Running tests...
Providing a value of "quit"...
Goodbye.
Providing a value of "bogus"...
You entered bogus.
Please help what is wrong on your statement?