Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial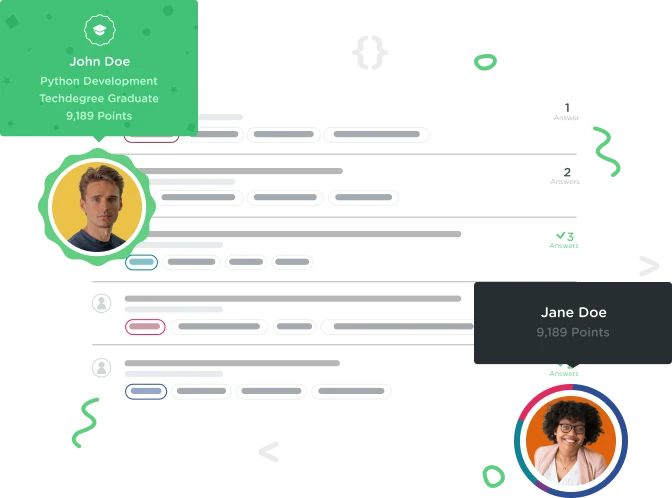
Karl Pupé
6,718 PointsVariable Scope - why Lilah, George and Lilah?
Hi Guys!
I have just watched the video and I am really sorry but I don't get the last part... I understand why Lilah is called because we are calling a function, I understand why on the 2nd part why Lilah then George because alert(person) exists in both the function variable and the global one but for the life of me, I can't figure out why it fires Lilah, George and then Lilah again...
I've checked out the answers in the community page and I am still baffled!
Maybe I'm slow but could someone break it down really slowly how it became Lilah, George and then Lilah again?
Thank you so much in advance!
1 Answer
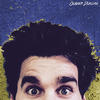
Oliver Duncan
16,642 PointsThis concept is crucial to coding with JavaScript, so it's worth spending some extra time on. Let's take another look at what's happening.
// ---- Global Scope ----
var person = 'George';
// ----------------------
// ---- Function Scope ----
function greeting() {
var person = 'Lilah';
console.log(person)
}
// ------------------------
greeting(); // creates new var person in function scope, prints 'Lilah';
console.log(person); // reads person in global scope, HAS NO ACCESS to 'Lilah'
greeting(); // once again creates var person in local scope, prints 'Lilah'
First, we declare a global variable person. It has global scope because it's not declared within a function, and is therefore accessible to the whole program.
Next, we write a function greeting() that declares a local variable person and logs it to the console. When we call greeting(), 'Lilah' will be printed to the console.
Okay, we're back in global scope, and we call greeting(). This function creates a new variable person, assigns it to the string 'Lilah', and logs it to the console.
Then, we call console.log(person). This is the crucial thing to understand -- when using person while in global scope, person equals 'George'. We no longer have access to to the local variable person, the one that equals 'Lilah'. The only way to access that variable is to call greeting().
Speaking of which, we call greeting() again. That function once again creates a new variable person, assigns it to the string 'Lilah', and prints it to the console. Thus we get 'Lilah', 'George', 'Lilah'.
Don't feel bad if you still don't get it, it's a complex topic and takes a while to fully understand. Try reading this, too, it's got some great examples.
Hope this helps!
Karl Pupé
6,718 PointsKarl Pupé
6,718 PointsThank you! Your description helped make the penny drop! Thank you!
I don't know if this will help anyone else but I came up with an analogy of SONY holding the rights to the Spiderman movie universe before he came to the MCU.
"Variables declared within a JavaScript function, become LOCAL to the function. Local variables have local scope: They can only be accessed within the function."
Think of SONY having the movie rights to 'Spiderman' before he joined the MCU. 'Spiderman' operated in his world and could not interact with any other Marvel Heroes so he was in a smaller universe. His actions had no baring to anything apart from his own movie universe.
"A global variable has global scope: All scripts and functions on a web page can access it. In other words, a function can change the value of a variable from the global scope. It's usually seen as the 'bigger' universe."
Think of the Global Function scope like the Marvel Cinematic Universe (MCU): it's got loads of characters which makes it 'bigger' movie universe and local variables behave like SONY releasing Spiderman back to the MCU: meaning although Spiderman was part of a smaller universe, he could came to effect the bigger one!
Thank you my friend!