Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial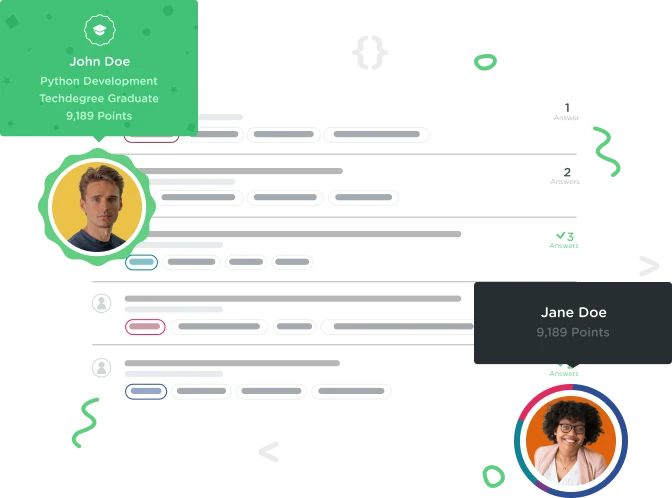
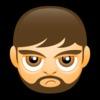
J D
318 PointsVariable Shadowing does not make sense to me.
Please take a look at these snippets:-
// (1)
var uniqueNum = 45;
function newNum(){
var uniqueNum = 55;
}
var uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 65 //output
/*==============================*/
// (2)
var uniqueNum = 45;
function newNum(){
var uniqueNum = 55;
}
uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 65 //output
/*==============================*/
// (3)
var uniqueNum = 45;
function newNum(){
uniqueNum = 55;
}
var uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 55 //output
/*==============================*/
// (4)
var uniqueNum = 45;
function newNum(){
uniqueNum = 55;
}
uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 55 //output
Question:-
I am kinda confused about variable assigned before, inside and outside function. Can somebody please help me with an explanation, as simple as possible on how they work. Like snippet 1 and 2 , are same I suppose and so are 3, and 4. But simply deleting var in-front of variable made such difference, thats what confused me.
2 Answers

Sarah Bradberry
7,115 Points// (1)
var uniqueNum = 45;
function newNum(){
var uniqueNum = 55;
}
var uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 65 //output
In this instance, you have a global variable uniqueNum with 45
uniqueNum within the function is 55. Because you used var here, it is a value that is only used within the function.
uniqueNum called after the function overwrites the global variable with a new value of 65. This is because this instance is not within a function.
Your code then calls the Newnum function but you're console.log calls uniqueNum without running a function so you get the value of uniqueNum that is last in your code. (because it overwrote the global variable with a new value)
// (2)
var uniqueNum = 45;
function newNum(){
var uniqueNum = 55;
}
uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 65 //output
Same as example 1
// (3)
var uniqueNum = 45;
function newNum(){
uniqueNum = 55;
}
var uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 55 //output
Because you didn't use var within the function your uniqueNum within the function has overwritten the global variable.
You have run the function immediately before your console.log so the uniqueNum within the variable just run is overwriting the value before it (65)
// (4)
var uniqueNum = 45;
function newNum(){
uniqueNum = 55;
}
uniqueNum = 65;
newNum();
console.log(uniqueNum);
> 55 //output
Same as 3
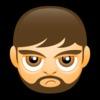
J D
318 PointsThanks a lot Sarah, it makes sense to me now.