Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial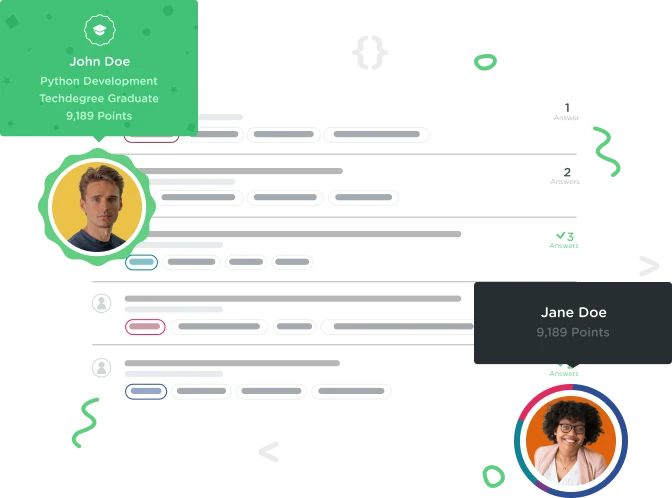
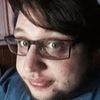
Unsubscribed User
3,721 PointsVariables not defined, but code still works?
Using what I have learned about functions, conditional statements and variables so far, I came up with the following solution for this challenge, which seems to work well.
I tried to use a function for each task - this also included using a function inside a function. I'm not sure of is if using so many functions best practice?
But the main question I have, is that even though I haven't defined any variables (e.g. var lower
or var upper
), the code still works?
Why is this?
//Random Number Generator
//User inputs data.
function generateInput(){
lower = parseInt(prompt ( "Type Starting Number:" ));
upper = parseInt(prompt ( "Type End Number:" ));
}
//Validate if user's input was a number.
function validateInput(){
if (isNaN(lower) || isNaN(upper)){
document.write("<p>Invalid Input. Please ensure only numbers are used.</p>");
throw new Error ("Input was not numeric");
}
}
//Generate random number
function generateNumber(){
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
//Generate output
function generateOutput(){
document.write("<p>A number between " + lower + " and " + upper + " is...</p>");
document.write("<p><strong>" + generateNumber(upper,lower) + "</strong></p>");
}
generateInput();
validateInput();
generateOutput();
Thanks, Berian
3 Answers
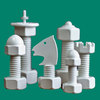
Steven Parker
231,269 PointsWhat you have is an "implicit global declaration".
Variables which are assigned without being declared are considered to be impliclty declared as globals.
While this may allow the code to work in many cases, it is considered a bad practice. It will also cause a syntax error when JavaScript is in the "strict" mode.
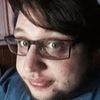
Unsubscribed User
3,721 PointsYeah I started watching Dave's lessons on Loops, and he states that many programmers declare variables to the top of their code, as you said before any functions. Perhaps I was jumping the gun a bit!
Thanks for your help, Steven. Much appreciated!
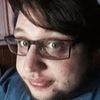
Unsubscribed User
3,721 PointsThank you! That makes makes sense!
What would be the best way to declare them has global variables in this instance?
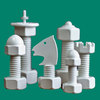
Steven Parker
231,269 PointsI think the most clear way to create global variables would be to declare them outside of and before any functions with the "var" keyword.