Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial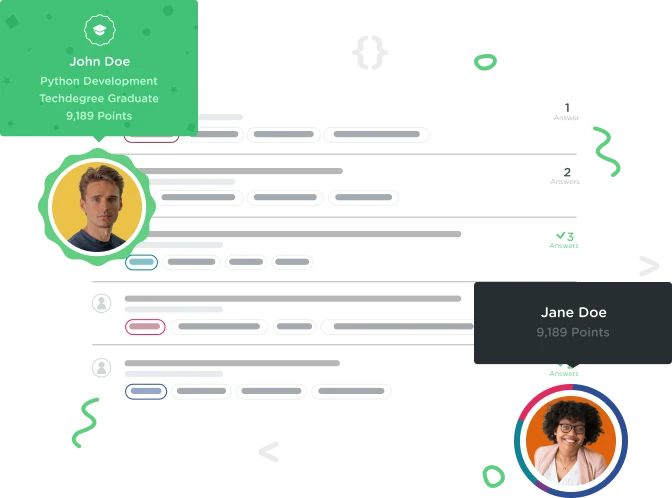

Russell Comer
26,102 PointsVariables or functions
I'm writing a little app basically just to practice on a small real world project and I'm curious if I should use variables instead of functions even if the functions are only a return statement.
const accountBalance = document.getElementById("accountBalance");
const stockPrice = document.getElementById("stockPrice");
// Returns shares that are allowed per trade
function sharesPerTrade() {
const amountPerTrade = accountBalance / 4;
return Math.floor(amountPerTrade / stockPrice);
}
// Returns amount allowed per trade
function amountPerTrade() {
return accountBalance / 4;
}
// Returns amount that should be calculated for limit and stop amounts
function getExitAmount(x) {
return ((accountBalance * x) / sharesPerTrade()).toFixed(2);
}
// Returns a proposed limit selling price
function limitPrice() {
return getExitAmount(.03) + stockPrice;
}
// Returns a proposed stop selling price
function stopPrice() {
return stockPrice - getExitAmount(.01);
}
// Returns possible profit from the trade
function possibleProfit() {
return (limitPrice() * sharesPerTrade()).toFixed(2);
}
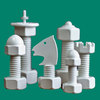
Steven Parker
231,269 PointsThat's not arrow function syntax. But this would be:
const amountPerTrade = () => accountBalance / 4;
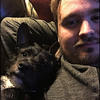
Matthew Long
28,407 PointsGood point. Steven. That's what happens when you comment on something at 3 in the morning.
1 Answer
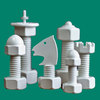
Steven Parker
231,269 PointsOne way to decide what to use is that variables store something, where functions do something.
For example, your function "amountPerTrade" returns the current value of "accountBalance" divide by 4. That's returning a value you get from doing something. If you had assigned a variable with this value, it continue to hold the same thing even if "accountBalance" changed later. But the function will always perform the calculation on the current value of "accountBalance".
Matthew Long
28,407 PointsMatthew Long
28,407 PointsMaybe use arrow functions? Might condense your code and improve readability.
const amountPerTrade => accountBalance / 4;