Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial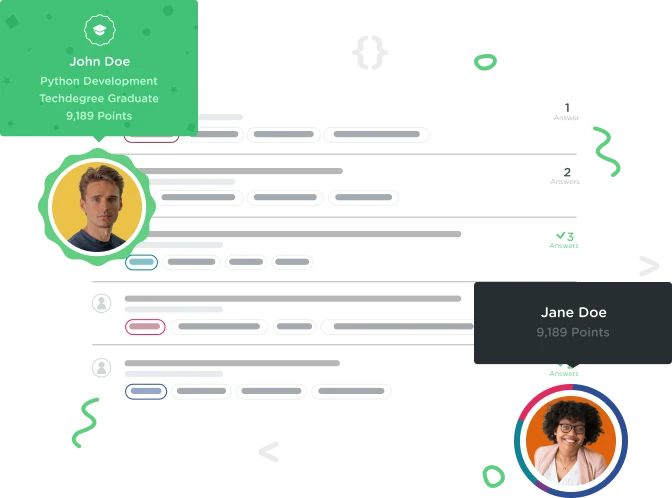
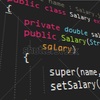
simon123123
1,291 PointsVarriables
How could I use this varriable in another class?
package com.example.help;
import android.app.Activity;
import android.os.Bundle;
public class MainActivity extends Activity {
public int counter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
counter = 0;
}
}
5 Answers
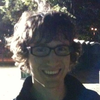
Ben Rubin
Courses Plus Student 14,658 PointsWhat do you mean use the variable in another class? What exactly are you trying to do?

Patrick Piwowarczyk
263 PointsI guess making a public method and returning the variable would work? Never tried it before.
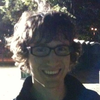
Ben Rubin
Courses Plus Student 14,658 PointsSince counter is a public variable, any other class can access it as long as that other class has a reference to the object of MainActivity. So you could do something like this
public class MainActivity extends Activity {
public int counter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
counter = 0;
MyOtherClass myObject = new MyOtherClass();
myObject.showCounterValue(this); // You have to pass in the current instance of MainActivity so that myObject has a reference to the MainActivity object
}
}
public class MyOtherClass {
public void showCounterValue(MainActivity myMainActivity)
{
log.i("LOG", "the value of counter is " + myMainActivity.counter); // You can access counter like this
}
}
Public variables and methods can be accessed by any class. You just have to have a reference to the object of that class first.
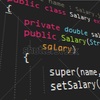
simon123123
1,291 PointsSorry I meant in another activity
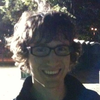
Ben Rubin
Courses Plus Student 14,658 PointsYou could use the putExtra method of the Intent class to pass data to your new activity when you start it.
public class MainActivity extends Activity {
public int counter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
counter = 0;
}
public void startNewActivity() {
Intent intent = new Intent(MainActivity.this, MyOtherActivity.this);
intent.putExtra("Counter Value", counter); // Add the counter value as extra data to the intent for the new activity
startActivity(intent);
}
}
public class MyOtherActivity extends Activity {
// Put your onCreate and other methods here
public showCounterValue() {
log.i("LOG", "the counter value is " + getIntExtra("Counter Value")); // Get the counter value that was added with putExtra()
}
}
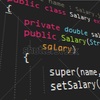
simon123123
1,291 PointsIt won't work for some reason
Isn't it supposed to say .class? Intent intent = new Intent(MainActivity.this, MyOtherActivity.class);
It still says: counter cannot be resolved to a variable
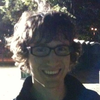
Ben Rubin
Courses Plus Student 14,658 PointsYeah, you're right about the .class. It shouldn't be saying counter cannot be resolved to a variable. Post your code.
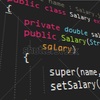
simon123123
1,291 PointsI'm on vacation right now I'll post it in a couple days
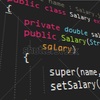
simon123123
1,291 PointsMain Activity
package com.example.help;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
public class MainActivity extends Activity {
public int counter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
counter = 0;
}
public void startNewActivity() {
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
intent.putExtra("Counter Value", counter); // Add the counter value as extra data to the intent for the new activity
startActivity(intent);
}
}
Second Activity
package com.example.help;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.widget.TextView;
public class SecondActivity extends Activity {
public TextView mtextview;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
mtextview = (TextView)findViewById(R.id.textview);
mtextview.setText(counter);
}
}
And I'm also not sure what the counter value is. Could you explain that to me?
simon123123
1,291 Pointssimon123123
1,291 PointsLike if I try to display this number in a different class. eg: Lets say I have a text view varriable in another class named mtextview and im trying to write the following line of code. mtextview.setText( StringOf.counter);
I would somehow need to import the counter varriable into the other class. How would I do this?