Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial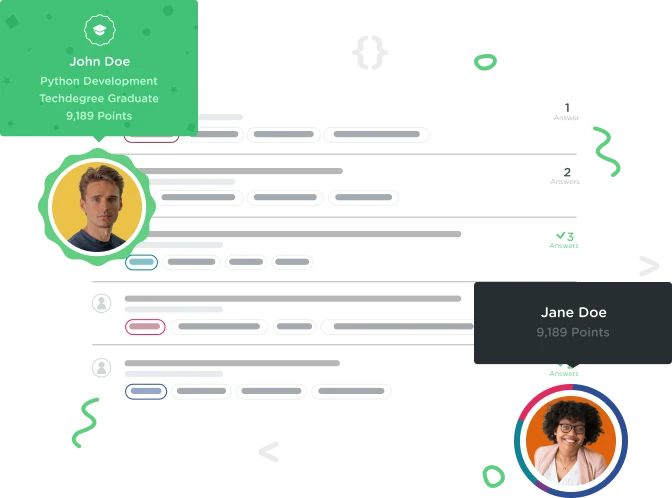
defectivebit
Courses Plus Student 3,771 PointsVehicle struct code challenge - no such module "UIKit"
So the code challenge says that the Vehicle struct needs to be initialized with numberOfWheels
equal to 4, numberOfDoors
equal to 4, and color
equal to the color blue. So to get the color I import UIKit so that I can use the UIColor class to make the color blue. But the code fails at the top saying that there is no such module UIKit. But how am I supposed to complete the code challenge without being able to use UIColor to get the color blue that it asks for?
import UIKit
struct Vehicle {
var numberOfWheels: Int
var numberOfDoors: Int
var color: UIColor
init() {
numberOfWheels = 4
numberOfDoors = 4
color = UIColor(red: 0.0, green: 0.0, blue: 1.0, alpha: 1.0)
}
}
2 Answers

Alvin Abia
Courses Plus Student 23,034 PointsHey there, I actually haven't gotten up to that specific code challenge yet but I have some possible answers for you based on the code you provided.
1) I see that you declared your var color as type String, what if you tried declaring it as type UIColor like this:
var color: UIColor
2) I also see that within your custom init method you access the blue color using RBGA values, what if you tried using UIColor's blueColor method like this:
color = UIColor.blueColor()
Lastly, I know that the above suggestions I provided do not tackle your issue with UIKit not being found as a module. Try removing it from your code challenge answer, and see what happens, perhaps the code challenge is already implicitly importing UIKit for you? I hope this answer helped, if none of the above suggestions work, please feel free to reply to me with any new error messages/information you receive and I'll try to help you further!
defectivebit
Courses Plus Student 3,771 PointsThought of implicit import but then it says UIColor isn't defined. I guess it does need to be declared as a UIColor though, thanks for the hint. Using RGBA shouldn't be an issue though, as it produces the same output.
EDIT: The var color: String
is actually a cut-and-paste straight from the challenge, so the challenge might be incorrect.