Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial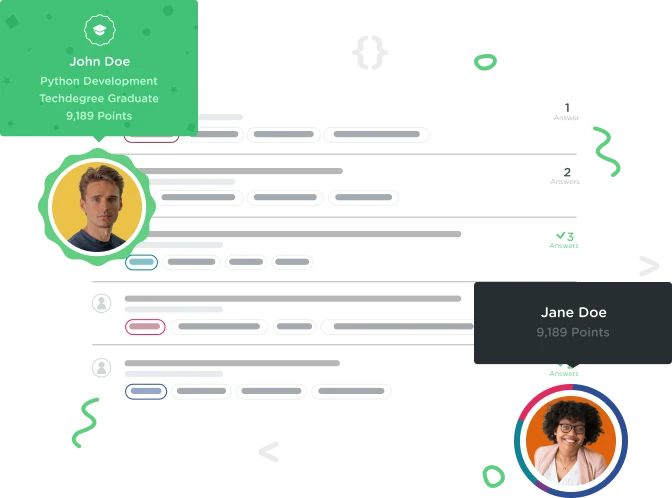

Robert Baucus
3,400 PointsVending Machine - using random.choice or random.shuffle and deleting the resulting choice/shuffle
#this version has the 'mystery' option which does a random.choice instead of pop. Still need to pop it...
import os
import random
def clear_screen():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
sodas = ["Cherry Coke Zero", "Crystal Pepsi", "DDP", "DrNut"]
chips = ["Funions", "Bugles", "Chex Mix", "Chili Cheese Fritos"]
candy = ["Fifth Avenue", "Abbazabba", "Big Hunk", "Sugar Daddy"]
while True:
choice = input(" Would you like a SODA, bag of CHIPS or some CANDY or MYSTERY ?!?!? (Q to quit)").lower()
clear_screen()
try:
if choice == 'soda':
snack = sodas.pop()
elif choice == 'chips':
snack = chips.pop()
elif choice == 'candy':
snack = candy.pop()
elif choice == 'q':
break
elif choice == 'mystery':
snack = (random.choice(sodas + chips + candy)).pop()
else:
print("What was that breh?")
continue
except IndexError:
print("We're all out of {}, sorry breh! Pick sumfingk else!".format(choice))
else:
print("Here's your bloody {}: {}, nice choice wanka".format(choice,snack))
I added an elif for the user to select 'mystery' which results in a random.choice from all the available items added together in one big list, so far so good. The problem results when I try to delete the result of this random choice/shuffle so it can't be selected again. The code as it is written here can not work because pop() won't work on a string, and I even tried putting a list() nested inside the pop() but that did not work either.
I looked online and the advice for this type of problem was to use shuffle() which yields alist, which should be able to be pop() 'ed , i.e.
snack = (random.shuffle(sodas + chips + candy)).pop()
but whenever I do that the result in the program is "None", what to do ?