Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial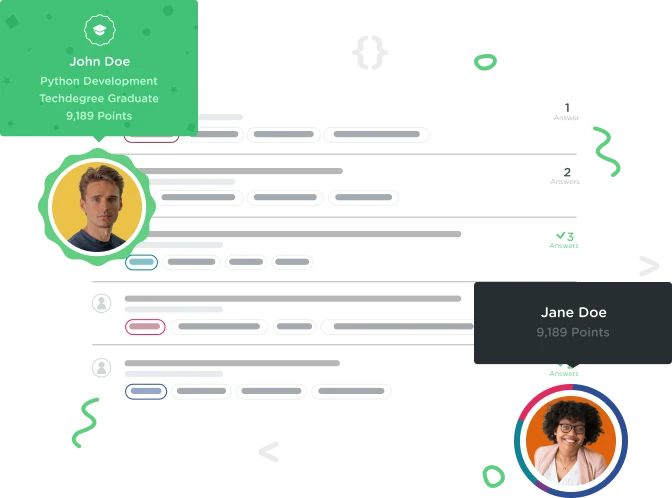

Alastair Herd
2,306 PointsVery strange compiler error
In ContactController.java, add a controller method that captures a URI request for "/contact", and adds a Contact object to a parameter ModelMap. The Contact object can have any id, firstName, lastName, and email that you like. But, it should added to the ModelMap using the key "contact". The method should render the view named "contact_detail".
I seem to be getting a compiler error on this code, but as far as I can see there isn't a problem with it at all!
Any help would be much appreciated,
Thank you!
Alastair
package com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.ui.ModelMap;
@Controller
public class ContactController {
@RequestMapping("/")
public String home() {
return "index";
}
@RequestMapping("/contact")
public String contactDetail(ModelMap modelMap) {
Contact contact = new Contact('1', "Alastair", "Herd", "adfa@email.com");
modelMap.put("contact",contact);
return "contact-details";
}
package com.teamtreehouse.contactmgr.model;
public class Contact {
private int id;
private String firstName;
private String lastName;
private String email;
public Contact() {}
public Contact(int id, String firstName, String lastName, String email) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public int getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getEmail() {
return email;
}
public void setId(int id) {
this.id = id;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setEmail(String email) {
this.email = email;
}
}
1 Answer

KRIS NIKOLAISEN
54,972 PointsDid you check the preview? If that is all you have you are missing a closing bracket for your class. After which you will receive: Are you sure that you returned the correct view name?
From the instructions and your post above: The method should render the view named "contact_detail".
Alastair Herd
2,306 PointsAlastair Herd
2,306 PointsThank you, it was the missing bracket and - instead of _.