Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial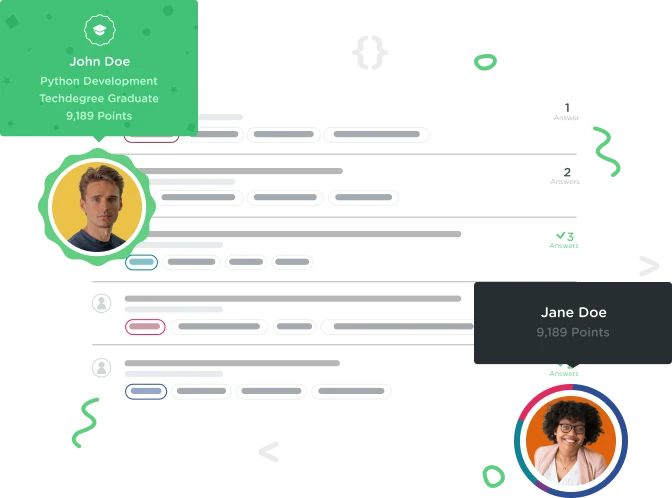

Wei Li
5,860 PointsVideoGamesRepository.cs(17,37): error CS0029: Cannot implicitly convert type `Treehouse.Models.VideoGame' to `Treehouse.
can't understand the error message. What is the right answer?
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public VideoGame[] GetVideoGames(int id)
{
VideoGame[] videoToReturn = null;
foreach (var _videoGame in _videoGames)
{
if(_videoGame.Id==id)
{
videoToReturn = _videoGame;
break;
}
}
return _videoGames;
}
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
2 Answers
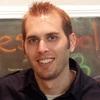
Rick Buffington
8,146 PointsFor Task 1 I think you are over thinking it. Because the _videoGames field is private, it can't be accessed by anything outside of this class. Basically all they are asking is for you to make a public access method. Because _videoGames is an array of VideoGame, we need to return a VideoGame array.
public VideoGame[] GetVideoGames()
{
return _videoGames;
}
For Task 2, things get a little more complex - and this code looks a little bit like what you were attempting in your code however they want a separate method for this code. So your iteration is close, just in the wrong method - they want a GetVideoGame method that returns 1 single video game. You are super close.
public VideoGame GetVideoGame(int id)
{
// Iterate through each video game in the _videoGames collection
foreach(var videoGame in _videoGames)
{
// If we find a match, go ahead and return it
if (videoGame.Id == id)
return videoGame;
}
// We didn't find a match so just return null
return null;
}
Hope that helps!

Edward Ries
7,388 PointsThe first one GetVideoGames is supposed to return the list of games and it's no supposed to take any parameters. The two important pieces to this method is that the return value has to have the array brackets "VideoGame[]" The second is the return statement which returns the array of games and has to match the return type which is VideoGame[].
public VideoGame[] GetVideoGames()
{
return _videoGames;
}
This method is meant to return a single game based on it's id. We set the return type to VideoGame and then we loop through the videos until we find an id that matches. Once we find the match we return the game. If we don't find a match then we return a null which is the absent of a value.
// TODO Add GetVideoGame method
public VideoGame GetVideoGame(int id)
{
foreach (var game in _videoGames)
{
if(game.Id==id)
{
return game;
}
}
return null;
}
Edward Ries
7,388 PointsEdward Ries
7,388 PointsLooks like I spent too much time typing. Looks like Rick and I had the same solution in mind. Code looks good Rick.