Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial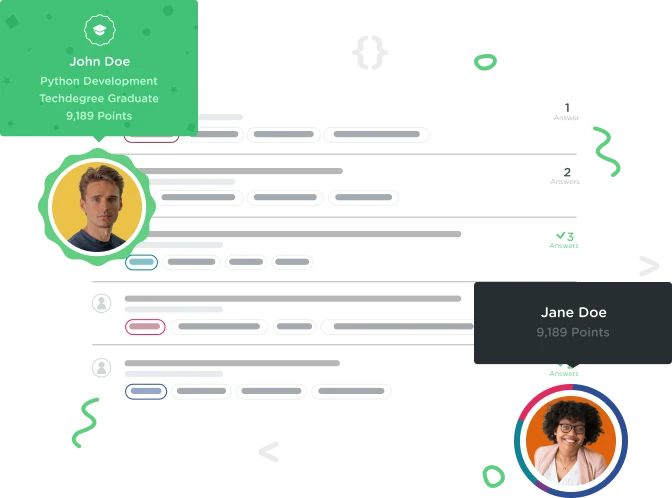

Dena G
441 PointsVideos not uploading locally in dev with Paperclip Gem in Rails. (Black screen with play button)
I'm able to upload my images however, when I try to upload videos it posts as a black page with a play button however, the video will not play. I'm not sure where I went wrong. My guess is perhaps the posts show page.
This may be an easy solution however, I'm new to Rails. Any help is greatly appreciated. Thank you.
Post Model
class Post < ActiveRecord::Base
belongs_to :user
has_attached_file :image, :styles => { :medium => "300x300>", :thumb => "100x100>" }
has_attached_file :video, :styles => { :medium => "300x300>", :thumb => "100x100>" }
validates_attachment_content_type :image, :content_type => ["video/mp4", "image/jpg", "image/jpeg", "image/png", "image/gif"]
validates :description, presence: true
validates :image, presence: false
validates :video, presence: false
end
AddAttachmentImageToPosts Model
class AddAttachmentImageToPosts < ActiveRecord::Migration
def self.up
change_table :posts do |t|
t.attachment :image
t.attachment :video
end
end
def self.down
drop_attached_file :posts, :image, :video
end
end
def change
create_table :videos do |t|
t.string :video_file_name
t.string :video_content_type
t.integer :video_file_size
t.datetime :video_updated_at
t.timestamps
end
end
Post _form
<div class="field">
<%= f.label :image %>
<%= f.file_field :image %>
</div>
<div class="field">
<%= f.label :video %>
<%= f.file_field :video %>
</div></br>
<div class="field">
<%= f.label :description %>
<%= f.text_field :description %>
</div>
Posts Show
<div class="row">
<div class="col-md-offset-4 col-med-8">
<div class="panel panel-default">
<div class="panel-heading center">
<%= image_tag @post.image.url(:medium) %>
<%= video_tag @post.video.url(:medium), controls: true, type: "video/mp4" %>
</div>
<div class="panel-body">
<p><%= @post.description %></p>
<p><strong><%= @post.user.name if @post.user %></strong></p>
<% if @post.user == current_user %>
<%= link_to edit_post_path(@post) do %>
<span class="glyphicon glyphicon-edit"></span>
Edit
<% end %>
<% end %>
<%= link_to 'Back', posts_path %>
</div>
</div>
3 Answers

Michael Wiss
19,233 PointsWhat happens if you use video_path instead of video_tag in your posts show?

Michael Wiss
19,233 PointsMaybe you need to try something like
<%= video_path @post.video.url(:medium), controls: true, type: "video/mp4" %>
Try searching http://stackoverflow.com/

Dena G
441 PointsThanks Michael. That actually helped however, now its just displaying a black screen with a play button however, the video will not play. Do I need a video player in order for this to work? I have checked stackoverflow however, I can't seem to pinpoint my problem.

Michael Wiss
19,233 PointsDo you use Chrome Dev tools? Can you inspect the "blank path?" I've never used paperclip with video before but I had a similar issue last week with images. Maybe you need to declare a file path for has_attached_file :video in the post model.
the paperclip documentation has this line for example:
```has_attached_file :avatar, :styles => { :medium => "300x300>", :thumb => "100x100>" }, :default_url => "/images/:style/missing.png" '''

Dena G
441 PointsThe path within Chrome Dev tools says that its a 404 Not Found. Request URL:http://0.0.0.0:3000/videos/medium/missing.png Request Method:GET Status Code:404 Not Found.
I added has_attached_file :video, :styles => { :medium => "300x300>", :thumb => "100x100>" } to the post model however, I still get a blank black screen. I think I may need a video player like ffmpeg. But I'm not sure. I really appreciate you helping me.
Dena G
441 PointsDena G
441 PointsHi Michael. It still shows up as blank but with '/videos/medium/missing.png' afterwards. It's odd because its a mov. video