Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial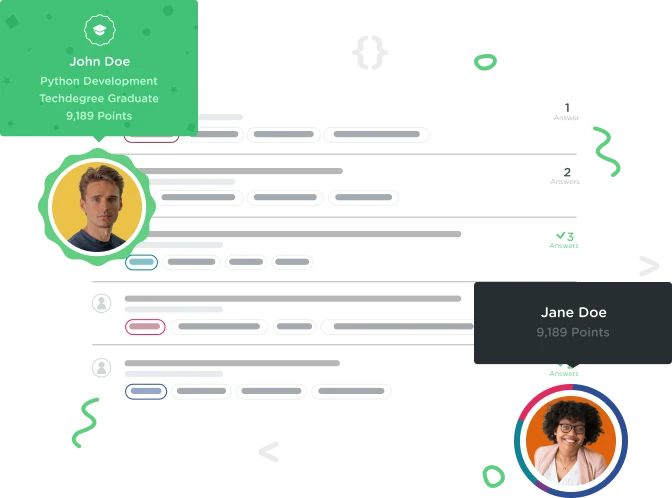

charlesdi
5,706 Pointsview holder is being changed to recycler view and iconImageView, temperatureLabel, dayLabel are not being recognize
The view holder is being changed to recyclerView which I assume is due to deprecation, but if I'm interpreting this correctly the getView method is unable to find the iconImageView, the temperatureLabel, and the dayLabel.
Code for reference:
package com.treehouse.android.stormy.adapters;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.treehouse.android.stormy.R;
import com.treehouse.android.stormy.weather.Day;
/**
* Created by charlesdirenzo on 8/1/17.
*/
//this will act as an adapter to pass information from the day object to the
public class dayAdapter extends BaseAdapter
{
private Context mContext;
private Day[] mDays;
public dayAdapter(Context context, Day[] days)
{
mContext = context;
mDays = days;
}
@Override
public int getCount()
{
return mDays.length;
}
@Override
public Object getItem(int position)
{
return mDays[position];
}
@Override
public long getItemId(int position)
{
return 0; //this will go unused. This can be used to tag items for easy reference.
}
@Override
public View getView(int position, View convertView, ViewGroup parent)
{
//viewHolder pattern will help us re-use resources and memory, power, and help the list be smoother.
//convert view is the view that we are reusing. if it is null it's new, and if not then it has data in it.
//layout inflater is an android object that takes xml layout and turns them into views in code that we can use.
RecyclerView.ViewHolder holder;
if (convertView == null)
{
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item,null);
holder = new RecyclerView.ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else
{
holder = (RecyclerView.ViewHolder) convertView.getTag();
}
}
return null;
}
private static class ViewHolder
{
ImageView iconImageView;
TextView temperatureLabel;
TextView dayLabel;
}
}