Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial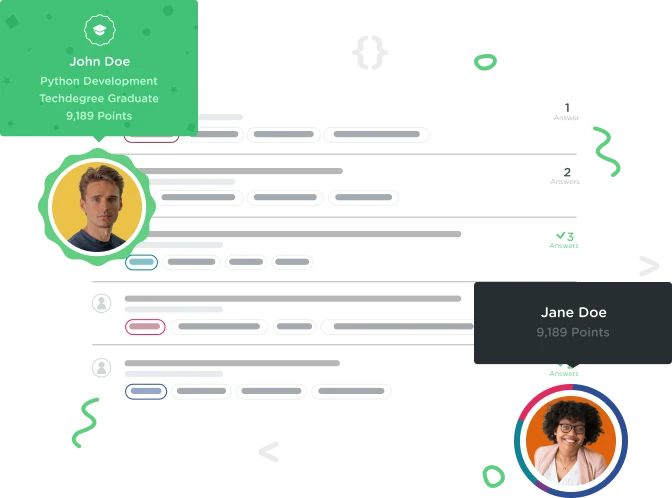
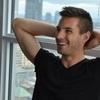
evanpavan
5,025 PointsVirtual methods code challenge - Not all code paths return a value
I'm getting the following error on compilation. I can't seem to figure it out as it appears that the conditional logic should always return true/false in one of the paths. I've tried a few variations and have ended up with the same error.
RepeatDetector.cs(5,27): error CS0161: `Treehouse.CodeChallenges.RepeatDetector.Scan(int[])': not all code paths return a value Compilation failed: 1 error(s), 0 warnings
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
if (sequence.Length < 2)
{
return false;
}
else
{
for(int i = 1; i < sequence.Length; i++)
{
if(sequence[i] == sequence[i-1])
{
return true;
}
else
{
return false;
}
}
}
}
}
}
2 Answers
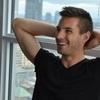
evanpavan
5,025 PointsYeah Brendan, you're right. C# didn't trust that I was catching all conditions within the If/else statement within the for loop. If I remove the else statement and add a catch all return of false as you suggested after the for loop then it compiles fine.
Old code that errors due to no return value
for(int i = 1; i < sequence.Length; ++i)
{
if(sequence[i] == sequence[i-1])
{
return true;
}
else
{
return false;
}
}
New code that compiles
for(int i = 1; i < sequence.Length; ++i)
{
if(sequence[i] == sequence[i-1])
{
return true;
}
}
return false;
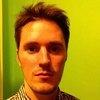
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI haven't dug that deep into the logic. But you can solve it by adding return false
, or whatever your default case is, at the end of your method. So in case none of these conditions are met and you haven't returned anything yet, you'll return that.
I'll take your word for it that the all the logic works and there's no case where it wouldn't return something. But maybe C# isn't that smart and it needs you to add some fail-safe at the end to make sure.