Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial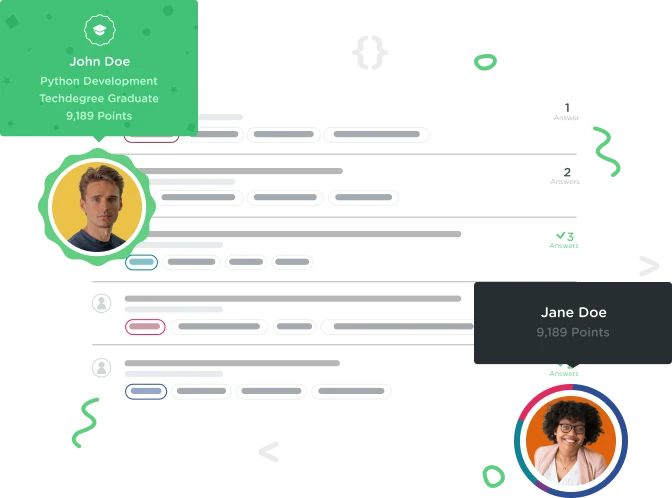

William Bok
6,842 PointsVM5944:13 Uncaught TypeError: Cannot read property '0' of undefined(…)
var userInput = ""; var numberOfCorrect = ""; var correctQuestions = []; var incorrectQuestions = []; var results = [ ["You got " + numberOfCorrect + "question(s) right."], ["You got these questions correct:"], [correctQuestions], ["You got these questions wrong:"], [incorrectQuestions] ];
var quiz = [ [ "What is 20 squared?" , "400" ], [ "What colour is the sky?" , "blue" ], [ "How many wheels on a bike?" , "2" ] ];
function answer( questions ) {
for ( i=0; i<questions.length; i+=1 ) { userInput = prompt ( questions [i][0] ); }
if ( userInput.toLowerCase() === questions [i][1] ) { numberOfCorrect+=1; correctQuestions[ questions [i][0] ]; } else { incorrectQuestions [ questions [i][0] ]; }
}
answer(quiz);
function print(message) { document.write (message); } print(results);
1 Answer

Benjamin Barslev Nielsen
18,958 PointsThe problem is with the for loop:
for ( i=0; i<=questions.length; i+=1 ) {
userInput = prompt ( questions [i][0] );
}
questions[questions.length] is undefined, so you should change the <= to <, such that the code is:
for ( i=0; i<questions.length; i+=1 ) {
userInput = prompt ( questions [i][0] );
}
William Bok
6,842 PointsWilliam Bok
6,842 PointsThanks for the quick response! I've changed the sign but still getting the error VM70:45 Uncaught TypeError: Cannot read property '1' of undefined(…)
Benjamin Barslev Nielsen
18,958 PointsBenjamin Barslev Nielsen
18,958 PointsOk, there is another problem, you do not use your userInput inside the loop:
The if-statement you have that checks the userinput is outside the loop, which means that it is first run, after all the iterations in the loop, i.e., when i = questions.length. Therefore you should put the if-statement inside the loop-body:
There is still a couple of problems with the code, but now the TypeError should be solved.