Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial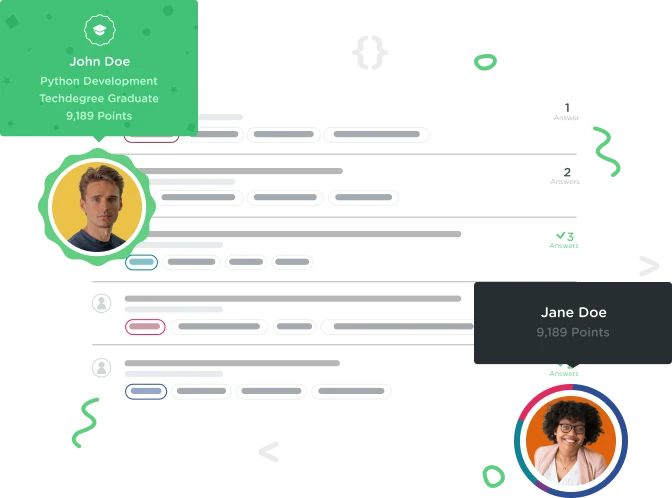
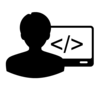
Abe Layee
8,378 PointsVoid type not allowed here
I am trying to print the room types. However, I am getting the error void type is not allowed here. I don't understand why? I have a method called public void printRoomTypes() and it job is to print the array.
import java.util.*;
import java.text.*;
import java.io.*;
import java.util.Arrays;
import javax.sql.rowset.spi.*;
class Hotel {
private String name;
private int rooms;
private int booked;
private String[] roomTypes = {"Twin","Double", "Suite"};
private boolean gym = false;
private boolean pool = false;
public Hotel() {}
public Hotel (String name, int rooms, int booked, boolean gym, boolean pool) {
super();
this.name = name;
this.rooms = rooms;
this.booked = booked;
this.gym = gym;
this.pool = pool;
}
public void setName (String Name) {
name = Name;
}
public String getName () {
return name;
}
public void Setroom(int Room){
rooms = Room;
}
public int getRoom (){
return rooms;
}
public void setBooked (int Booked){
booked = Booked;
}
public int getBooked (){
return booked;
}
public int checkAvailability (){
return this.rooms - this.booked;
}
public boolean hasGym() {
return true;
}
public boolean hasPool() {
return false;
}
public void printRoomTypes() {
for (int i = 0; i < roomTypes.length; i++) {
System.out.println( " Room sizes: " + roomTypes[i]); //this doesn't work too
}
}
public static void main(String[] args){
Hotel hotel = new Hotel();
hotel.setName("Hamilton");
hotel.Setroom(40);
hotel.setBooked(22);
System.out.println("Hotel name: " + hotel.getName());
System.out.println("Total rooms: " + hotel.getRoom());
System.out.println("Total rooms booked: " + hotel.getBooked());
System.out.println("Rooms available " + hotel.checkAvailability() + "\n");
System.out.println(hotel.printRoomTypes()); // this doesn't work
System.out.println("......................\n");
Hotel newHotel = new Hotel();
newHotel.setName(" Sheraton");
newHotel.Setroom(100);
newHotel.setBooked(95);
System.out.println("Hotel name: " + newHotel.getName());
System.out.println("Total rooms: " + newHotel.getRoom());
System.out.println("Total rooms booked: " + newHotel.getBooked());
System.out.println("Rooms available " + newHotel.checkAvailability());
}
}
1 Answer
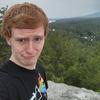
Brendon Butler
4,254 PointsWhen you use the println
method, anything inside of that has to be a data type. Inside your println
method (10 lines in your main method), you're calling the printRoomTypes
method which returns void. If it returned a string, int, etc. it would work properly. The compiler sees it as you trying to print nothing which causes an error.
I looked at your printRoomTypes
method, and it looks as if it does all the printing for you. Here's your solution:
// replace this line
System.out.println(hotel.printRoomTypes()); // this doesn't work
// with this line
hotel.printRoomTypes();
Abe Layee
8,378 PointsAbe Layee
8,378 Pointsty