Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial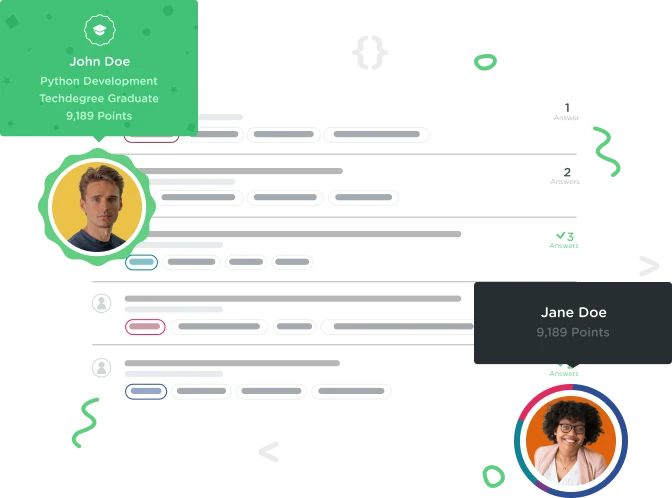

ghaith omar
16,395 Pointsvowel challenge
Hi all I am trying to do the vowel challenge in python course. I wrote a code but didn't work in the challenge, but its working when I try it. The challenge about removing any vowel on the world. this is my code :
def disemvowel(word):
x = ""
word = list(word.lower())
while True:
if "a" in word:
word.remove("a")
if "e" in word:
word.remove("e")
if "o" in word:
word.remove("o")
if "i" in word:
word.remove("i")
if "u" in word:
word.remove("u")
if "a" in word or "o" in word or "e" in word or "i" in word or "u" in word:
continue
else:
break
for gn in word:
x +=gn
word = x
return word
def disemvowel(word):
x = ""
word = list(word.lower())
while True:
if "a" in word:
word.remove("a")
if "e" in word:
word.remove("e")
if "o" in word:
word.remove("o")
if "i" in word:
word.remove("i")
if "u" in word:
word.remove("u")
if "a" in word or "o" in word or "e" in word or "i" in word or "u" in word:
continue
else:
break
for gn in word:
x +=gn
word = x
return word
5 Answers
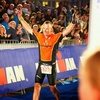
Steve Hunter
57,712 PointsAh - you're not preserved the case of the word sent in.
You can get round that by testing if letter.lower() not in vowel
, rather than changing the whole word to lowercase.
So, lose word_lower
completely and loop through word
(not word_lower
) using letter
, as you have done. Then change your if
statement to the above and that should work, I think.

eg3
2,681 PointsHI all ! I wrote this and tested it on repl.it. But the Teamtreehouse system doesn't accept it. What's wrong in here? Any one want to help?
def disemvowel(word):
word = list(word)
remove_this = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
no_vowels = []
for i in word:
if i in remove_this:
word.remove(i)
else:
no_vowels.append(i)
no_vowels = "".join(no_vowels)
return (no_vowels)
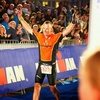
Steve Hunter
57,712 PointsIf you remove from the thing you are iterating over, you cause real problems.
Let's iterate over my name. On the first pass, we're on 'S', which we then remove for whatever reason. We start with:
1 2 3 4 5
S T E V E
After removing 'S' we are left with:
1 2 3 4
T E V E
But the loop will continue to iteration number 2, so you bypass the 'T' completely and go straight to 'E'.
That's why it's easier to build a string of the characters you want rather than remove the characters you don't want.
Make sense?
Steve.
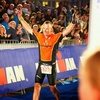
Steve Hunter
57,712 PointsYou've actually done what you need to - there's more lines than required and there's one that causing the issue. If you just comment out your remove
line and replace it with a pass
your code works.
def disemvowel(word):
word = list(word)
remove_this = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
no_vowels = []
for i in word:
if i in remove_this:
pass
#word.remove(i)
else:
no_vowels.append(i)
no_vowels = "".join(no_vowels)
return (no_vowels)
However, you can be more brief:
def disemvowel(word):
output = ""
vowels = ['a', 'e', 'i', 'o', 'u']
for l in word:
if l.lower() not in vowels:
output += l
return output
Steve.
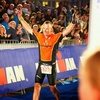
Steve Hunter
57,712 PointsHi there,
One issue is that you've changed the case of word
so your returned string will not be the same as the input string, minus vowels.
Try looking at this a little differently. Let's create an empty string to output/return at the end of the method. Let's create a list holding all the vowels, too. Then, use a for
loop to iterate over the parameter, word
.
At each iteration, see if the lower case version of the current letter is not in the list of vowels. If it isn't, add it to the output string.
At the end of the loop - return that string.
Give that a try and let me know how you get on.
Steve.

ghaith omar
16,395 PointsThanks for the fast reply. I try this method and didn't work, this the new code
def disemvowel(word):
x = ""
vowel = ["a","o","i","u","e"]
word_lower = list(word.lower())
for letter in word_lower:
if letter not in vowel:
x += letter
return x
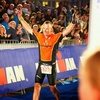
Steve Hunter
57,712 PointsLooks good - let me see what's up with it ...

Ismail KOÇ
1,748 PointsIf you want output words are upper and lower, you can use this code:
def disemvowel(word):
x = ""
word = list(word)
ret_list = word # add this for do not damage word list for remove vowels.
vowels = ['a','e','i','o','u','A','E','I','O','U']
for i in word:
if i in vowels:
ret_list.remove(i)
for j in ret_list:
x += j # return string
return x
print(disemvowel('abceFiGu'))
I added vowels = ['a','e','i','o','u','A','E','I','O','U'] because deleting lower and upper vowels and return of the function only deleting vowels. (this is alternative, you can use this if you want)
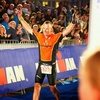
Steve Hunter
57,712 PointsOr you can just test if i.lower() in vowels:
which makes your list shorter too.

ghaith omar
16,395 PointsThanks for the answer.

Ismail KOÇ
1,748 PointsOr you can just test
if i.lower() in vowels:
which makes your list shorter too.
yeah you are right (how can i add other emojis in texts)
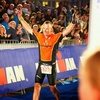
Steve Hunter
57,712 Points
eg3
2,681 PointsWow steve thank you very much for taking time to answer my question :-) !
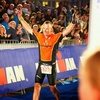
Steve Hunter
57,712 PointsNo problem!
ghaith omar
16,395 Pointsghaith omar
16,395 PointsThanks it worked, when I removed .lower() from word, and add it to letter.lower() thanks for help
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsGlad you got it sorted.