Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial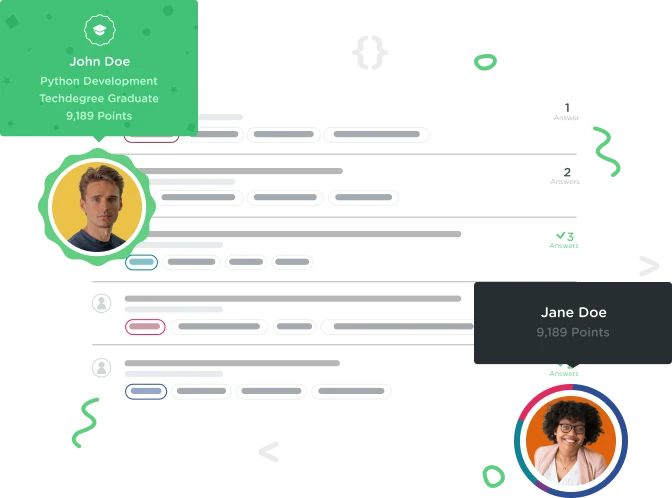

zahid hasan
3,600 PointsWarning: Failed propType: Application: prop type `players` is invalid; it must be a function, usually from React.PropTyp
After the compilation I see that error but I dont understand why players must be function?
3 Answers

nico dev
20,364 PointsHi Joshua Weber,
I know it's a little late, but hopefully not yet too late. :)
Maybe you already found out, but just in case, the thing in your code that's giving you that error is a misspelling in your isRequired
for the players
prop of the Application
component, almost at the end of your code.
To be more specific, right here:
Application.propTypes = {
title: React.PropTypes.string,
players: React.PropTypes.arrayOf(React.PropTypes.shape({
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
id: React.PropTypes.number.isRequired,
})).isRequred, // should say isRequired
};
I think the rest is perfect. Worked perfect when I tried it, just correcting that one.
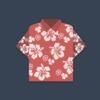
Joshua Weber
7,411 Pointshey zahid hasan how did you fix the error? I'm getting the same error you mentioned.
var PLAYERS = [
{
name: "My Name",
score: 31,
id: 1,
},
{
name: "Her Name",
score: 142,
id: 2,
},
{
name: "His Name",
score: 75,
id: 3,
},
{
name: "Your Name",
score: 62,
id: 4,
},
]
function Header(props) {
return (
<div className="header">
<h1>{props.title}</h1>
</div>
);
}
Header.propTypes = {
title: React.PropTypes.string.isRequired,
};
function Counter(props) {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> {props.score} </div>
<button className="counter-action increment"> + </button>
</div>
);
}
Counter.propTypes = {
score: React.PropTypes.number.isRequired,
}
function Player(props) {
return (
<div className="player">
<div className="player-name">
{props.name}
</div>
<div className="player-score">
<Counter score={props.score} />
</div>
</div>
);
}
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
}
function Application(props) {
return(
<div className="scoreboard">
<Header title={props.title} />
<div className="players">
{props.players.map(function(player) {
return <Player name={player.name} score={player.score} key={player.id} />
})}
</div>
</div>
);
}
Application.propTypes = {
title: React.PropTypes.string,
players: React.PropTypes.arrayOf(React.PropTypes.shape({
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
id: React.PropTypes.number.isRequired,
})).isRequred,
};
Application.defaultProps = {
title: "Scoreboard",
}
ReactDOM.render(<Application players={PLAYERS}/>, document.getElementById('container'));

Jon Wood
9,884 PointsCan't tell for sure since there's no code, but the component must be specifying what types the props are. It's probably saying that it requires a function, but you may be passing in something else like maybe a string.

zahid hasan
3,600 PointsI have fixed it. Thank you.