Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial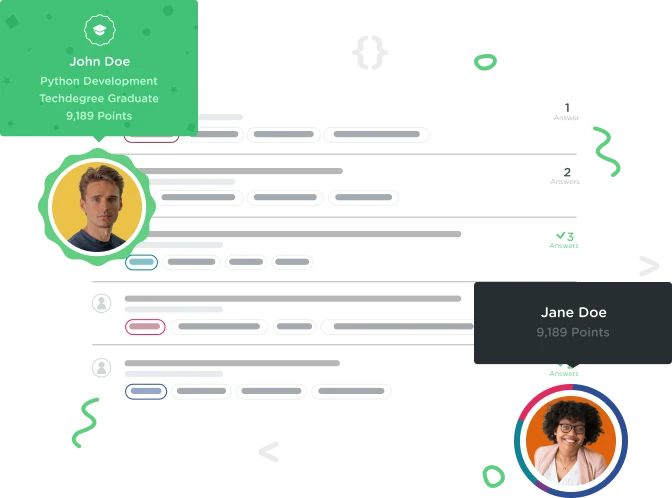

Denny Louis
10,885 PointsWarning: You should not use <Route component> and <Route children> in the same route; <Route children> will be ignored
In the console I get the message "Warning: You should not use <Route component> and <Route children> in the same route; <Route children> will be ignored".
What would be the best way to nest the routes since it creates this conflict in React Router v4?
6 Answers

Jonathan Dewitt
8,101 PointsAdding my original answer to this one --
I got around this first issue by changing my render function accordingly:
// Render
render((
<Router>
<App>
<Route exact={true} path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/courses" component={Courses} />
<Route path="/teachers" component={Teachers} />
</App>
</Router>
), document.getElementById('root'));
I had some trouble with that next lesson as well (I just did it today) -- it seems like React has changed the ability to nest routes as taught in the videos. To include the sub-route, I researched and used a <Switch> tag within Courses.js file.
Also, it seems NavLink is now part of react-router-dom, so you can just include the module instead of making your own.
Here's what I came up with:
Courses.js - underneath the closing </ul></div>
<Redirect from="/courses/" to="/courses/html" />
<Switch>
<Route path="/courses/html" component={HTML} />
<Route path="/courses/css" component={CSS} />
<Route path="/courses/javascript" component={JavaScript} />
</Switch>
Be sure to import the three components into your file, and also be sure to include the needed modules in the react-router-dom import (NavLink, Redirect, Switch)

Denny Louis
10,885 PointsThank you! Got it working. They really need to update the videos for this.
Thanks again
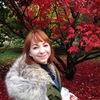
Sarah Ellis
959 PointsI found an easier way. You don't need to use the Redirect in the Courses.js. In the Router.js you can set the Switch like so:
// Libs
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
// Components
import App from './components/App';
import Home from './components/Home';
import About from './components/About';
import Teachers from './components/Teachers';
import Courses from './components/Courses';
// Components -- Courses
import HTML from './components/courses/HTML';
import CSS from './components/courses/CSS';
import JavaScript from './components/courses/JavaScript';
// Routes
// contain all routes into a variable to be imported into index.js
const routes = (
<Router>
{/* If path is / then load the Home component */}
<App>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/teachers" component={Teachers} />
<Courses>
<Route path="/courses/html" component={HTML} />
<Route path="/courses/css" component={CSS} />
<Route path="/courses/javascript" component={JavaScript} />
</Courses>
</Switch>
</App>
</Router>
);
export default routes;
This is all using V4

Guil Hernandez
Treehouse TeacherHi Darren Anderson,
Now that React Router v4 has shipped (and stable), I'm currently working on a course refresh. Stay tuned. :)
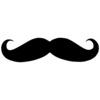
hum4n01d
25,493 PointsPhew!

Darren Anderson
274 PointsThis really needs to be updated. At this point these videos are incredibly frustrating to follow. Feels like I'm stopping in every video to try to find a solution to code not working.

Guil Hernandez
Treehouse TeacherWe released the new course two weeks ago: React Router 4 Basics. :)
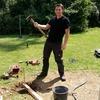
Nejc Vukovic
Full Stack JavaScript Techdegree Graduate 51,574 PointsI stomped on the same problem. In the meantime I'll have to checkout other courses - a lot for JS :D
Guil Hernandez can't wait for the updated Router course!

Andrew Sams
4,859 PointsETA?
Denny Louis
10,885 PointsDenny Louis
10,885 PointsHey Jonathan,
Thanks for that, that worked for this part of the routing. Can I ask what you did for the routing on the Courses page that is part of the challenge later on in the course?
Also, it won't let me mark your answer as correct because I think you did it as a comment rather than as an answer.
Thanks!