Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial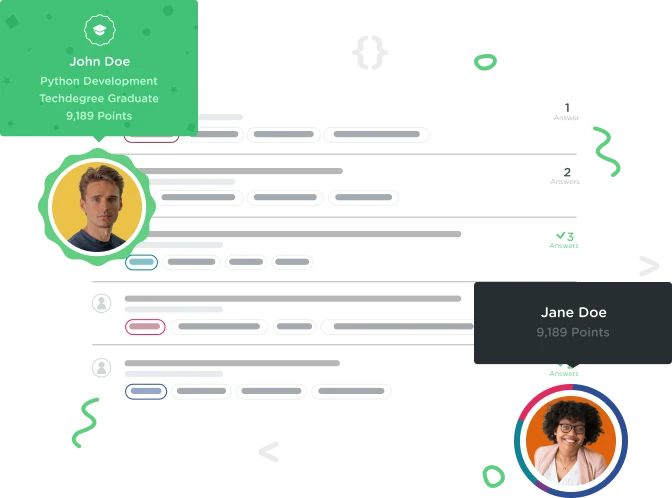
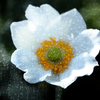
ellie adam
26,377 PointsWarrior 4 of 4
from character import Character
class Warrior(Character): weapon = "sword" pass def rage(self): self.attack_limit = 20
def str(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
from character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
MY error message is "try again"
7 Answers
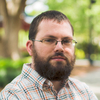
Kenneth Love
Treehouse Guest TeacherOh, hey, I couldn't see this on my phone well enough to spot the error. So, few things:
- Indentation, like I said on Twitter. But you said you got that so, on to number 2.
- You're calling
rage
withrage()
. That would be fine ifrage
was a function and not a method. You need to callself.rage()
. You also wantself.weapon
instead of justweapon
, since these things belong to the instance. -
string
doesn't exist when you do.format()
. Not sure what you're expecting to come in there. Maybeself.__class__.__str__()
? It's probably easier and safer to just do"Warrior"
.
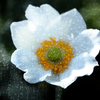
ellie adam
26,377 PointsI tried this code and got same error
def __str__(self):
string = "{}, <{}>, <{}>".format(string, self.weapon.__str__, self.rage.__str__())
return string
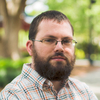
Kenneth Love
Treehouse Guest Teacherdef __str__(self):
string = "{}, <{}>, <{}>".format(
string,
self.weapon.__str__,
self.rage.__str__()
)
return string
self.weapon
is already a string, so you don't need to use .__str__
on it. That's not going to get you a string anyway, it's going to get a reference to a bound function (aka method). Similar error for the self.rage.__str_()
.
And what's your thinking behind the string
that is your first argument to format
?
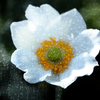
ellie adam
26,377 PointsYour answer looks perfect but I get same message even though I copy paste it.
from character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "{}, <{}>, <{}>".format(
string,
self.weapon.__str__,
self.rage.__str__()
)
return string
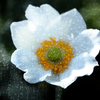
ellie adam
26,377 PointsIt is keep saying try again.
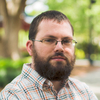
Kenneth Love
Treehouse Guest TeacherWell, mine is exactly the same as yours, just syntax highlighted and slight format changes.
You haven't answered my question, though. Can you walk me through each line? What are you expecting to happen at each step of the __str__
method?
I could just give you the answer, of course, but I want to know what I missed that get you confused so I can fix it for you and other students. You're really really close.
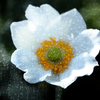
ellie adam
26,377 Points@Kenneth Love
The question is asking me in this part of challenge level 4 of 4.
warrior is converted to string. " "
string is = to "warrior, weapon, rage"
update your class to produce this string ("") using values from instance
those will be string, self.weapon.str, self.rage.str()
-
when string return, it will return the values from instance
still can't pass.
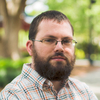
Kenneth Love
Treehouse Guest TeacherThere's nothing in the challenge about a variable named string
or about using the __str__
method for either weapon
or rage
.
Let's pretend I have a Warrior
instance named Furiosa.
furiosa = Warrior()
How would I get to Furiosa's attack_limit
?
How would I get to her weapon
?
Now, can you use those answers to fix the __str__
method in your class?
(And you don't need the string
variable. Just put the word Warrior
in your string before the two {}
s for weapon
and attack_limit
)
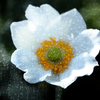
ellie adam
26,377 Pointsfrom character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "Warrior, {}, {}".format(weapon, rage())
return string
error: try again
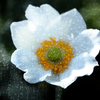
ellie adam
26,377 Pointsdef __str__(self):
string = "Warrior, {}, {}".format(
self.weapon,
self.attack_limit
)
return string
Finally this code passed.
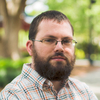
Kenneth Love
Treehouse Guest TeacherThat's it! Congratulations! Good job pushing through.
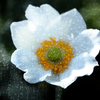
ellie adam
26,377 PointsThanks Kenneth!
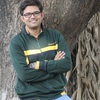
Prashant chaudhari
Courses Plus Student 24,499 Pointsgot it thanks

MUZ140953 Herbert Samuriwo
7,929 Pointsdef str(self): string = "Warrior, {}, {}".format( self.weapon, self.attack_limit ) return string
THIS ONE WORKS FOR ME
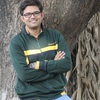
Prashant chaudhari
Courses Plus Student 24,499 Pointsthis work for me.
def str(self): string = "Warrior, {}, {}".format(self.weapon, self.rage()) return string