Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial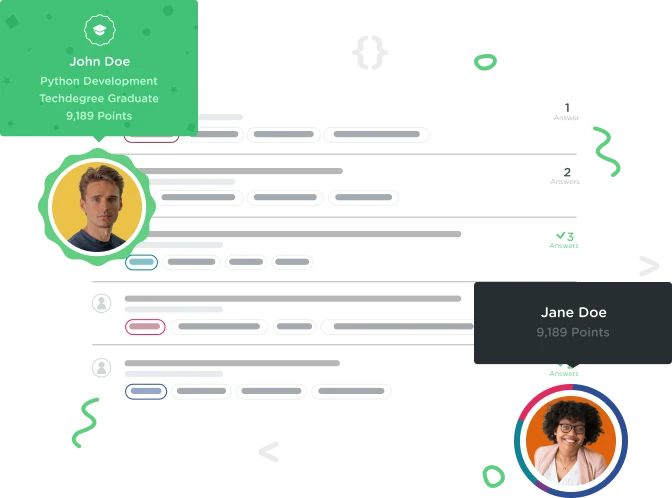
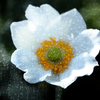
ellie adam
26,377 Pointswarrior string Q 4 of 4
from character import Character
class Warrior(Character): weapon = "sword" pass def rage(self): self.attack_limit = 20
def __str__(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
Error: task 1 no longer pass
from character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
3 Answers

James Simshaw
28,738 PointsIn your first example, you don't need the string parameter in the format. Also, since self.weapon is a string already, you don't need to add on the .str. For the last(second now) parameter, you don't want to have the warrior rage, but you want to print out the current attack_limit, so self.attack_limit

James Simshaw
28,738 PointsHello,
I'm not sure if it got mangled in the copy and paste, but you have one indentation error which is causing that error. In Python, indentation is important. For example, it tells python when to end a block of code. So you'll want to unindent your def statement to look like
from character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
That will at least get rid of the indentation error. From here you need to remove the < and > from your string. The instructions were using those to tell you that you need to fill those in with certain variables. So you'd want to change your string to "Warrior, {}, {}" where you're filling in the first blank with the weapon variable and the second with the attack limit variable. Make sure you use the proper syntax to reference the member variables attached to that object. Please let us know if this helps or if you need more assistance. and we can help you further. If you do need more assistance, please provide your updated code so that we can help you from where you are at.
Edit The pass that you have written in as well could probably be removed without any issue.
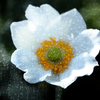
ellie adam
26,377 Pointsfrom character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "Warrior, {}, {}".format(
string,
self.weapon.__str__,
self.rage.__str__()
)
return string
I get error message; "try again"
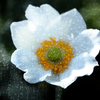
ellie adam
26,377 PointsI also tried this too.
from character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "{}, {}, {}".format(
string,
self.weapon.__str__,
self.rage.__str__()
)
return string
error: try again
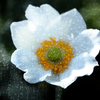
ellie adam
26,377 Pointsfrom character import Character
class Warrior(Character):
weapon = "sword"
pass
def rage(self):
self.attack_limit = 20
def __str__(self):
string = "Warrior, {}, {}".format(weapon, rage())
return string
error: try again
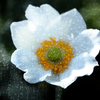
ellie adam
26,377 Points def __str__(self):
string = "Warrior, {}, {}".format(
weapon,
rage())
return string
error: try again
ellie adam
26,377 Pointsellie adam
26,377 Pointserror: try again
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsYour rage function was correct to begin with. From
all you need to do is change the
to
ellie adam
26,377 Pointsellie adam
26,377 PointsThank you so much James!
ok I will do it.
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsYou're welcome. Though you might want to mention in your other thread that you also have this thread and that things are solved so that those helping you there would know at what stage you are at.