Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial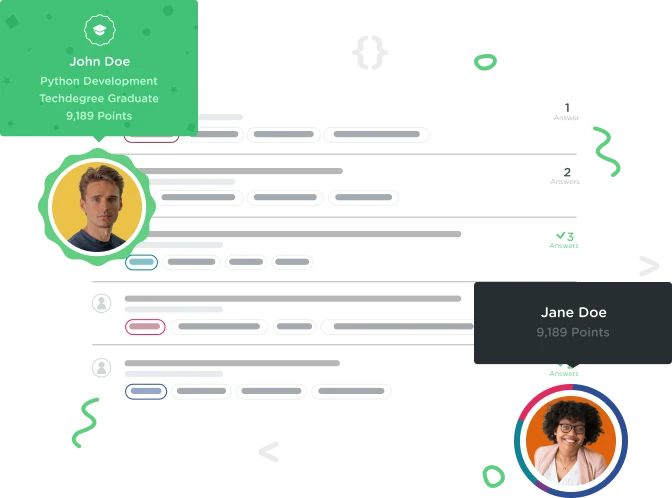
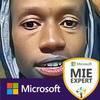
Tawanda Parayangiwa
2,614 Pointswas i suppose to do this ? confused
was i suppose to do this ? confused
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
public static List<WeatherForecast> DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
var serializer = new JsonSerializer();
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
}
}
using System;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
[JsonProperty(PropertyName = "weather_station_id")]
public string WeatherStationId { get; set; }
[JsonProperty(PropertyName = "time_of_day")]
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature { get; set; }
[JsonProperty(PropertyName = "precipitation_chance")]
public double PrecipitationChance { get; set; }
[JsonProperty(PropertyName = "precipitation_amount")]
public double PrecipitationAmount { get; set; }
public static SerializeWeatherForecasts(List<WeatherForecast>)
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
1 Answer
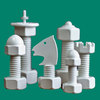
Steven Parker
231,269 PointsWhat was it you did so far? It's not obvious at first glance.
In particular, the instructions for the first task say "Create a new public static method named SerializeWeatherForecasts
...", but there doesn't seem to be a method by that name in the code shown above.