Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial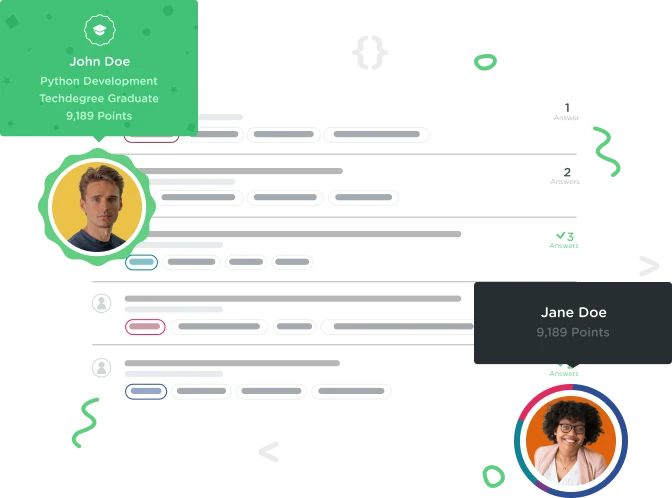
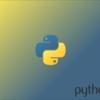
Kevin Faust
15,353 Pointswas this the right way to do the challenge?
Challenge Task 1 of 1
Create a function named sillycase that takes a string and returns that string with the first half lowercased and the last half uppercased.
This was pretty hard to come up with and I feel like there is an easier way
def sillycase(s):
new_list = []
half = round(len(s) / 2)
other_half = len(s) - half
lower = s[:half].lower()
new_list.extend(lower)
reverse = s[::-1]
reverse_upper = reverse[:other_half]
upper = reverse_upper[::-1].upper()
new_list.extend(upper)
return "".join(new_list)
2 Answers
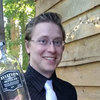
Evan Demaris
64,262 PointsHi Kevin,
I wouldn't say that was the most efficient way to pass the Challenge, but it's "right" if it passes and results in what the Challenge was asking. I've included a condensed version of the answer below, though.
def sillycase(string):
return (string[:round(len(string)/2)].lower() + string[round(len(string)/2):].upper())
Hope that helps, let me know if you have any questions!
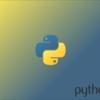
Kevin Faust
15,353 Pointsi dont understand how the second part works. Also, if the string was something with 9 letters such as "treehouse", would it still work?
Example with "treehouse":
for the first part: 9/2 = 4.5 which gets rounded to 5. So it would look like -> string[0:5].lower() which means letters 0 to 4 ("treeh") would be lowercased
for the second part: 9/2 = 4.5 which gets rounded to 5. So it would look like -> string[5:end].upper() which means letters 5 to the end ("ouse") would be capitalized
and then we add the two strings together
I ended up understanding the solution while I was writing down what i didnt understand. ill just leave that explanation above ^^ in case others are wondering as well
Much simpler solution. thanks
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Points4.5 gets rounded to 4. So in this example only "tree" would be lowercased which agrees with the example in the code comments.
In python 3 whenever a number is halfway between 2 choices it will round to the even choice. i.e. 4 instead of 5
5.5 would be rounded up to 6 because that's the even choice.
So in this challenge, the middle character for odd length strings will sometimes be placed in the 2nd half and sometimes in the first half.
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsBut doesn't that mean the "h" will never get affected? If4.5 gets rounded to 4 -> string[0:4].lower() means "tree" will get lower casedvice versa, string[4:0].upper() would mean "house" would get uppercaseed
I understood it again while writing down what i didnt understand lol
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsWorth mentioning; in Python 2.7 (not used in the Workspaces here, but still fairly common, especially if you're working with code that's been used for a while), round() functions as you'd expect it to with 4.5 rounding to 5, 5.5 to 6, etc.