Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial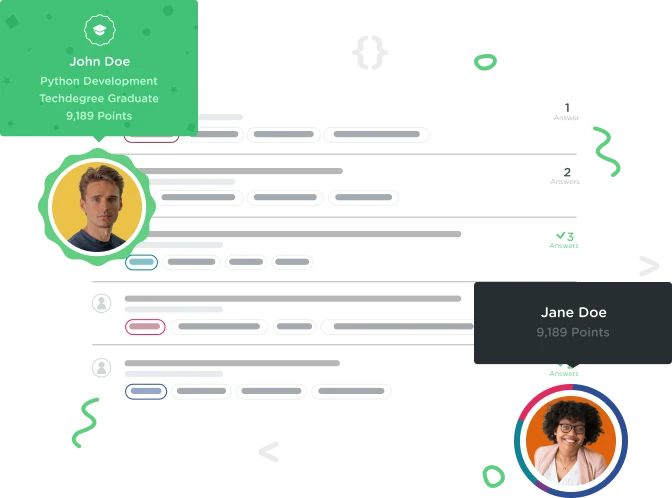
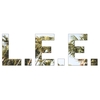
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsWay to store "first_name", ... with ruby on rails 4
To be able to save the first_name, last_name and profile_name, you can do the following steps: 1) Be sure to have added first_name, last_name and profile_name to the user migration file
class DeviseCreateUsers < ActiveRecord::Migration
def change
create_table(:users) do |t|
t.string :first_name
t.string :last_name
t.string :profile_name
end
end
end
2) Create a new file called registrations_controller.rb in the app/controllers directory. In this file u add the following code
class RegistrationsController < Devise::RegistrationsController
private
def sign_up_params
params.require(:user).permit(:first_name, :last_name, :profile_name, :email, :password, :password_confirmation)
end
def account_update_params
params.require(:user).permit(:first_name, :last_name, :profile_name, :email, :password, :password_confirmation, :current_password)
end
end
3) Finally you have to change your routes.rb file
Rails.application.routes.draw do
devise_for :users, :controllers => { registrations: 'registrations' }
resources :statuses
root to: 'statuses#index'
end
This should normally fix the problem
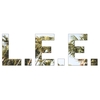
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsYes thats's a lot easier to read :) thanks
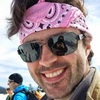
Jack McDowell
37,797 PointsI tried this and sadly it didn't work for me. As someone who did the ODOT app first, this app seemed like a great refresher, but now I'm stuck with a "NameError in Statuses#index" error. Any ideas? Here is my GitHub fork in case anyone can help
2 Answers
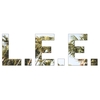
Lee Geertsen
Java Web Development Techdegree Student 16,749 PointsI had that problem too. It was because of an old status that didn't have a user associated with it. Just run
rake db:reset
in your console and than it should work :)
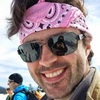
Jack McDowell
37,797 PointsRight, it has to do with the status being poorly created. When I run rake reset, or drop and migrate it will work, until I make a new status. Then, it goes back to being broken =/
Edit: (On the Devise GitHub page)[https://github.com/plataformatec/devise/wiki/How-To:-Allow-users-to-sign-in-using-their-username-or-email-address] they talk about changing the application controller to allow the extra fields, might this be an answer? I'll try tinkering with it later, it seems that the problem has to do with the added strong authentication and the lack of default "sanitized" fields in Devise. Why they didn't include f.name/l.name/u.name as defaults is beyond me...

Kris Chery
935 PointsJust sent you a note.
Jacob Moyle
6,150 PointsJacob Moyle
6,150 PointsBe sure to preview your markup before submitting. It'll make it a lot easier for other people to read: