Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial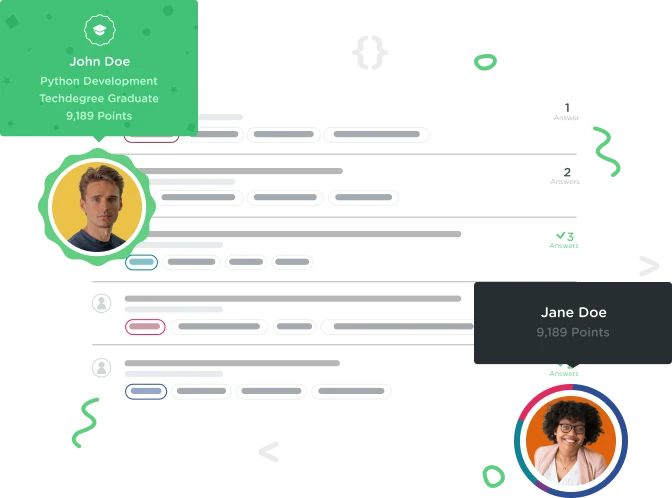

Grim Fandango
5,804 PointsWays to make this code more efficient? :)
Hi,
I think this is working as it should, but I am looking for ways to make this more streamlined. I suspect that there is a way to cover scores 1-2 and 3-4 in one else if statement that I've forgotten along the way!
Any feedback most welcome :)
console.log("Program start");
// answers to the questions
var answer1 = "PARIS";
var answer2 = "BLUE";
var answer3 = "DOWN";
var answer4 = "TWO";
var answer5 = "YELLOW";
//score counter
var score = 0;
//questions
var question1 = prompt("What is the captial of France?");
if (question1.toUpperCase() === answer1) {
alert("Correct!");
score += 1;
} else {
alert("Wrong."); }
var question2 = prompt("What colour is the sky?");
if (question2.toUpperCase() === answer2) {
alert("Correct!");
score += 1;
} else {
alert("Wrong."); }
var question3 = prompt("What is the opposite of up?");
if (question3.toUpperCase() === answer3) {
alert("Correct!");
score += 1;
} else {
alert("Wrong."); }
var question4 = prompt("How many eyes do humans tend to have?");
if (question4.toUpperCase() === answer4) {
alert("Correct!");
score += 1;
} else {
alert("Wrong."); }
var question5 = prompt("What color is yellow?");
if (question5.toUpperCase() === answer5) {
alert("Correct!");
score += 1;
} else {
alert("Wrong."); }
// write score to screen
if (score === 5) {
document.write("Flawless! Your total score was " + score + "! You get a GOLD CROWN!");
}
else if (score === 4) {
document.write("Nice work! Your total score was " + score + "! You get a SILVER CROWN!");
}
else if (score === 3) {
document.write("Nice work! Your total score was " + score + "! You get a SILVER CROWN!");
}
else if (score === 2) {
document.write("Hmm; not bad. Your total score was " + score + ". You get a BRONZE CROWN!");
}
else if (score === 1) {
document.write("At least you tried. Your total score was " + score + ". You get a BRONZE CROWN!");
}
else {
document.write("Sorry, your total score was " + score + ". You GET NOTHING");
}
console.log("Program end");
Thanks
3 Answers

Maximillian Fox
Courses Plus Student 9,236 PointsThe code looks very well laid out - so the next thing to do is look at the DRY programming - or 'Don't Repeat Yourself' way of programming.
I can see one block in your code that repeats, which is this part:
if (question3.toUpperCase() === answer3) {
alert("Correct!");
score += 1;
} else {
alert("Wrong.");
}
Why don't we try and put this into a function. I'm not going to type the whole code out (as this is something that you'll benefit from sorting yourself) but here's the gist of it
function isAnwerCorrect(question, answer){
//If the user's input in uppercase matches the answer, add 1 to score and return Correct! in an alert box
//Else, return Wrong in an alert box.
}
Then you can call your function for each question like this:
var question1 = prompt("What is the captial of France?");
isAnswerCorrect(question1, answer1);
and watch your code shrink but do the same job :)

Grim Fandango
5,804 PointsExcellent, thanks for your help! I definitely need to get my head around functions; they seem magical!

Maximillian Fox
Courses Plus Student 9,236 PointsThey are definitely a good thing to learn, and will save you LOTS of time especially on larger projects where you may need to repeat a spot of code in several places.
The simplest way to try functions is to do simple maths ones and work up from there. It may seem more long winded for really simple addition etc, but it will teach you the ins and outs.
// The function
function addNumbers(number1 + number2){
return number1 + number2; //The return statement makes sure the function chucks out the value.
}
// Calling it -- your variable names for the inputs don't have to be the same as the arguments.
var num1 = 3;
var num2 = 4;
var total = addNumbers(num1, num2);
alert(total); //Shows 7
You can also do functions on strings, arrays, objects, anything...
Silly example... but it will show you use of while loops which you'll see/have seen
function justAddFoo(string, numOfTimes){
var foo = ' foo!';
var i = 0;
while(i < numOfTimes){
string += foo; // "string += foo" is short for "string = string + foo"
i += 1 //Increments i up by 1
}
return string;
}
var myString = "aaaa...";
var myFoo = justAddFoo(myString, 3);
alert(myFoo); // aaaa... foo! foo! foo!
Note - code may have errors - just written off the cuff :)

Grim Fandango
5,804 PointsThere's a lot of food for though here - really appreciate all your input; thanks :)
Maximillian Fox
Courses Plus Student 9,236 PointsMaximillian Fox
Courses Plus Student 9,236 PointsYou can also create less if statements at the end, so instead of
The reason that the >2 will be fine is because if the score was not 5, the else if is triggered, so by doing >2, you check for === 3 and === 4 in one statement.