Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial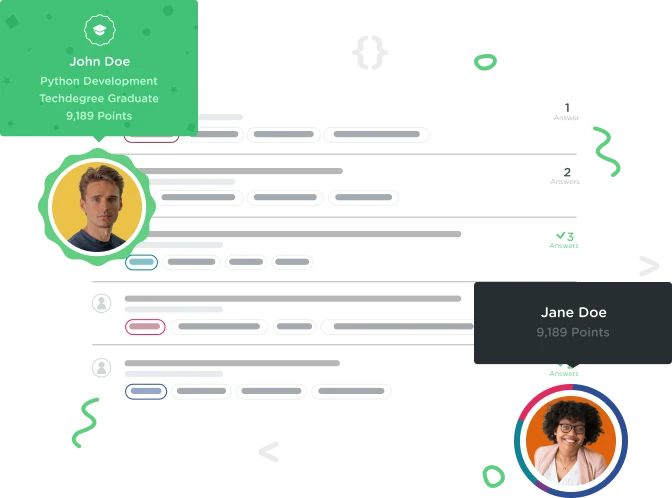

Andrew Merrick
20,151 PointsWays to reverse a string in Python?
This is in reference to the Stringcases challenge in the Tuples section of Python collections: http://teamtreehouse.com/library/stringcases
I was able to pass but I was wondering if there's a built in method to reverse a string, i.e. my_string.reverse()?
my_string.reverse() and my_string.reversed()
did not work since, I believe, they only apply to lists. I found out another way using slicing, but is there a method to reverse a string in Python?
3 Answers

Stone Preston
42,016 PointsI think you can use
''.join(reversed(my_string))
if you dont want to use slicing
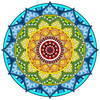
Pierre Guiglion
11,583 PointsYou can also use:
my_string[::-1]
It actually "slices" your string, going through all of it, by steps of -1. The slicing syntax is:
[<start>:<end>:<step>]

Andrew Merrick
20,151 PointsThanks, Pierre. That's actually how I was able to pass the challenge. Thanks for the help.
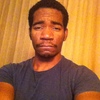
Stephen Whitfield
16,771 Points
Andrew Merrick
20,151 PointsStephen:
I tried using the my_string.reverse() (which I originally found on the link you provided), i.e.:
my_string = "hello world!"
my_string.reverse()
but I received a Traceback error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'str' object has no attribute 'reverse'
I think the list.reverse() only works for lists and not strings, but I could be mistaken. I think the:
"".join(reverse(my_string))
and
my_string[::-1]
are the better options. Thanks for the help, though!
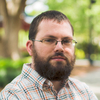
Kenneth Love
Treehouse Guest TeacherWe're using Python 3, though. Strings haven't changed much, but be careful about using Python 2 docs.
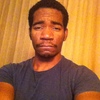
Stephen Whitfield
16,771 PointsAndrew Merrick Apologies. That's correct. .reverse() only works on lists. I was actually suggesting that you split the string into letters, reverse that list, and join them back together all in one line of code. my_string[::-1] is a far better solution though.
Andrew Merrick
20,151 PointsAndrew Merrick
20,151 PointsThanks, Stone! That worked as well.