Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial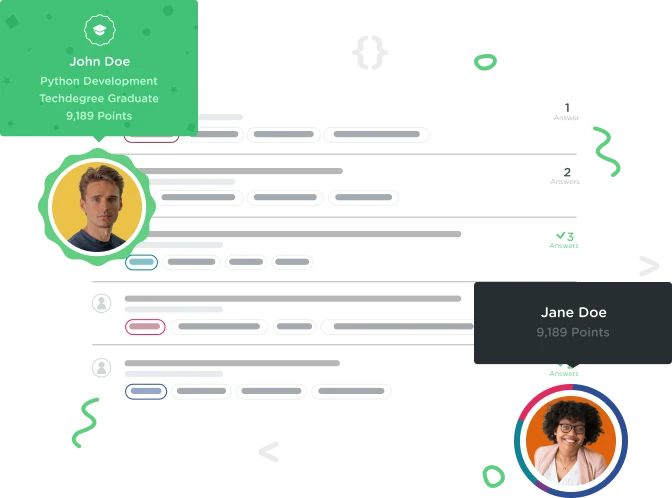

Blessing Chiwaura
18,037 PointsWe can't stop the query in product_detail for the Reviews that belong to a Product, but we can move it up so it's done B
We can't stop the query in product_detail for the Reviews that belong to a Product, but we can move it up so it's done at the same time as fetching the product.
First, though, change the product = get_object_or_404(...) to be a try and except combo that raises an Http404 exception if the Product doesn't exist.
Bummer! Didn't get a 200.; Didn't get a 404.
help thanx in advance
import datetime
from django.db.models import Q, Avg
from django.http import Http404
from django.shortcuts import render, get_object_or_404
from . import models
def good_reviews(request):
reviews = models.Review.objects.filter(rating__gte=3)
return render(request, 'products/reviews.html', {'reviews': reviews})
def recent_reviews(request):
six_months_ago = datetime.datetime.today() - datetime.timedelta(days=180)
reviews = models.Review.objects.exclude(created_at__lt=six_months_ago)
return render(request, 'products/reviews.html', {'reviews': reviews})
def product_detail(request, pk):
try:
product = get_object(models.Product, pk=pk)
except Product.DoesNotExist:
raise Http404('')
four_weeks_ago = datetime.datetime.today() - datetime.timedelta(weeks=4)
reviews = product.review_set.filter(Q(rating__gte=8)|Q(created_at__gte=four_weeks_ago)).order_by('-created_at').all()
return render(request, 'products/product_detail.html', {'product': product, 'reviews': reviews})
def product_list(request):
products = models.Product.objects.annotate(avg_rating=Avg('review__rating')).all()
return render(request, 'products/product_list.html', {'products': products})
2 Answers
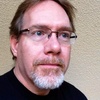
Chris Freeman
Treehouse Moderator 68,423 PointsThe is no function get_object()
. The challenge wants you to use the previously learned method using models.Product.objects.get(pk=pk)
.
The exception error class should include the models
module name: models.Product.DoesNotExist
. Product
does not exist outside of the models
namespace.
This should get you to Task 2. Good Luck!!!

Blessing Chiwaura
18,037 PointsThank you it passes