Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial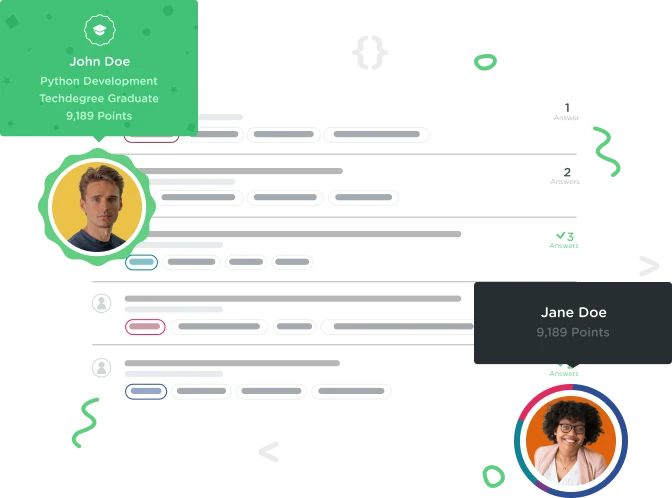
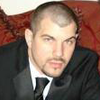
Gary Calhoun
10,317 PointsWe created a variable for <ol> but not <li>?
I was just trying to understand why we didn't create a variable for the <li> tag but we did for the <ol> tag or is just because we have to have a variable to print so it could of been anything?
function printList( list) {
var listHTML = '<ol>';
for ( var i = 0; i < list.length; i += 1 ) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
2 Answers
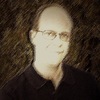
Jason Anders
Treehouse Moderator 145,860 PointsHi Gary. I think the purpose of creating the variable is to store the concatenation of the completed string. In real life, you wouldn't have had to assign the ol tag to the variable to have it still work.
You could have declared an empty string variable and just started the concatenating with the ol tag. For example:
function printList( list) {
var listHTML ""; //declare an empty string
for ( var i = 0; i < list.length; i += 1 ) {
listHTML = '<ol>' += '<li>' + list[i] + '</li>'; //added the <ol> tag to the concatenated string
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
So, it's not that one tag was declared and not the other, it was just a programming choice to start the variable with the ol tag already declared. In my opinion, neither way is better than the other in syntax or DRY programming; however, the one with the ol tag declared in the variable would be easier to figure out if you were looking at the code and not the original programmer.
Make sense? I hope so.
Keep Coding! :)
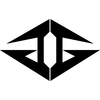
Julian Gutierrez
19,201 PointsIn this example the variable isn't only holding the ordered list "ol". It's being used to store the concatenated html being produced by running through the loop. The variable will end up storing something similar to the below html without the comments.
<ol> <!-- Initial variable declaration -->
<li>List item 1</li> <!-- First iteration of loop -->
<li>List item 2</li> <!-- Second iteration of loop -->
<li>List item 3</li> <!-- Third iteration of loop -->
</ol> <!-- Added after the escaping the for loop -->
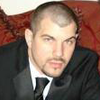
Gary Calhoun
10,317 PointsThanks for the example!
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsThanks yes makes perfect sense now:)
Diego Caballero de Alba
11,507 PointsDiego Caballero de Alba
11,507 PointsThe only problem with this is that the <ol> tag is inside the for loop and it has to be outside!
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsAh, yes Diego, you are correct. I guess I was too tied up in declared and empty variables that I wasn't paying attention to the loop and its purpose.
Good Catch! :)