Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial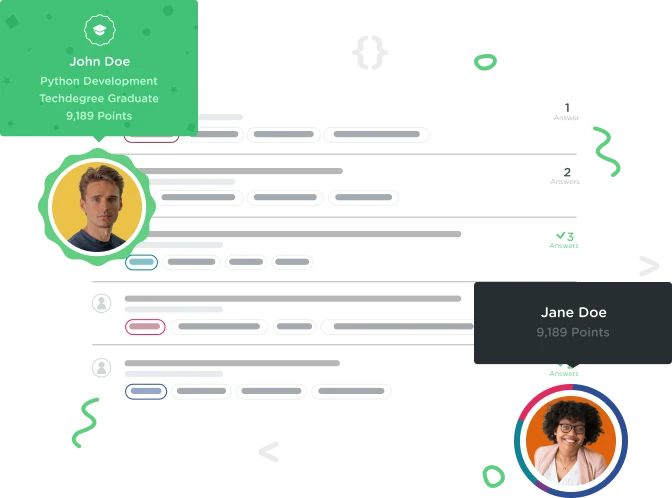
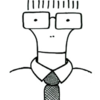
Gene Higgins
16,582 PointsWeather app code for node.js Basics extra credit
var http = require('http');
var https = require('https');
var forecastApiKey = "your api key here";
var googleApiKey = "your api key here";
var lat = "";
var long = "";
var zipcode = process.argv[2];
// print out error messages
var printError = function (error) {
console.error("error received");
console.error(error.message);
}
var geoRequest = https.get("https://maps.googleapis.com/maps/api/geocode/json?address="+zipcode+"&key="+googleApiKey, function(response){
var body = "";
response.on('data', function(chunk){
body += chunk;
});
response.on('end', function(){
if (response.statusCode === 200){
try {
// Parse the data
var location = JSON.parse(body);
lat = location.results[0].geometry.location.lat;
long = location.results[0].geometry.location.lng;
//call the weather API
getWeather(lat,long);
} catch (error) {
// Parse Error
printError(error);
}
} else {
// Status Code Error
printError({message:"There was an error getting the lat/long for "+zipcode+". ("+http.STATUS_CODES[response.statusCode]+")"});
}
});});
// Connect to the weather API
var getWeather = function(lat,long){
var request = https.get("https://api.forecast.io/forecast/"+forecastApiKey+"/"+lat+","+long,function(response){
var body = "";
// Read the data in
response.on('data', function (chunk) {
body += chunk;
});
response.on('end', function(){
if (response.statusCode === 200){
try {
// Parse the data
var weather = JSON.parse(body);
// Print the data
console.log(weather.hourly.summary);
} catch (error) {
// Parse Error
printError(error);
}
} else {
// Status Code Error
printError({message:"There was an error getting the weather for "+zipcode+". ("+http.STATUS_CODES[response.statusCode]+")"});
}
});
});
}
// connection error
geoRequest.on('error', printError);
3 Answers

Riley Gelwicks
6,214 PointsHey,
I wouldn't publish your API keys anywhere. Just simple OpSec.
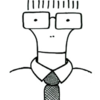
Gene Higgins
16,582 Pointser, right...I must've posted twice because I was fairly positive I took those out. Huh.
Thanks for the heads up.

Riley Gelwicks
6,214 PointsNo problem, I think treehouse is a relatively safe community but you just never know. Especially when your tinfoil hat is a tight as mine.
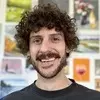
Joseph Guerra
20,674 PointsNice job using the google maps api to get latitude and longitude for the zip!