Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial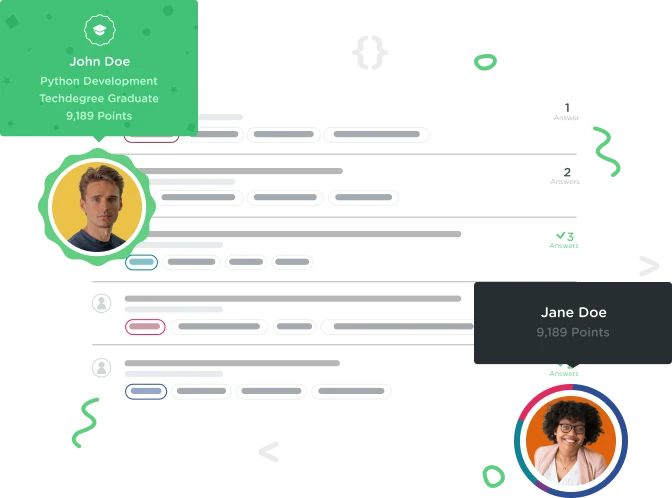
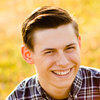
Caleb Kleveter
Treehouse Moderator 37,862 PointsWeather values in the detail view are just showing default values! Help!
Are you having an issue with Stormy showing the default weather values in the detail view for the temperature, rain, and humidity labels? Here is how I fixed it.
I you look at Pasan's if-statement for the labels, you will notice they all have to pass for the values to show (not great, right?). What you have to do is check them all separately. Now I don't want the default values to show if nothing is available (or the app to crash), so I set the values to N/A. Here is my code to replace the if-statement in the detail view configureView
method:
if let lowTemp = weather.minTemperature {
lowTemperatureLabel?.text = "\(lowTemp)ΒΊ"
} else {
lowTemperatureLabel?.text = "N/A"
}
if let highTemp = weather.maxTemperature {
highTemperatureLabel?.text = "\(highTemp)ΒΊ"
} else {
highTemperatureLabel?.text = "N/A"
}
if let rain = weather.percipChance {
precipitationLabel?.text = "\(rain)%"
} else {
precipitationLabel?.text = "N/A"
}
if let humidity = weather.humidity {
humidityLabel?.text = "\(humidity)%"
} else {
humidityLabel?.text = "N/A"
}
That is what fixed it for me.

Beste Erdem
114 Pointsr home , if you're using the downloaded files, the key for minTemperature was misspelled in DailyWeather.swift. You should correct is as dailyWeatherDict["temperatureMin"]. Also don't forget to call configureView() in viewDidLoad(). Optional chaining works fine you don't have to do the check one by one.
2 Answers
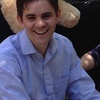
Thomas McCracken
13,591 PointsI had misspelled the key "precipProbability" in my DailyWeather struct. This caused all the optionals to be the default values for high and low temp, humidity, and rain because they were all defined in the optional chain which failed to nil for the rain.
One way I discovered this is to get rid of the option chain and unwrap each weather property at a time like so:
if let lowTemp = weather.minTemperature{
self.lowTemperatureLabel?.text = "\(lowTemp)ΒΊ"
}
if let highTemp = weather.maxTemperature {
self.highTemperatureLabel?.text = "\(highTemp)ΒΊ"
}
if let rain = weather.precipChance {
self.precipitationLabel?.text = "\(rain)%"
}
if let humidity = weather.humidity {
self.humidityLabel?.text = "\(humidity)%"
}
This way you can see which one of the properties on the weather object is failing to be assigned the correct value. Hope this helps!

r home
Courses Plus Student 108 PointsIt does work....but not for lowTemperature value...its always N/A for my app...any Idea why?
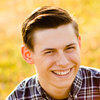
Caleb Kleveter
Treehouse Moderator 37,862 PointsI would like to see your code, but my issue was a miss spelled data point. Copy and paste the name of the data point from the Dark Sky API docs and see if that fixes it.
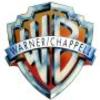
WCM Operations
Courses Plus Student 15,102 PointsI was having the same problem. I checked my DailyWeather.swift file and found that in the init method I had a typo when setting the minTemperature:
minTemperature = dailyWeatherDict["temparatureMin"] as? Int
should be
minTemperature = dailyWeatherDict["temperatureMin"] as? Int
It's unlikely that you have the exact same problem, but it might be worth checking that file to insure there are no typos.
r home
Courses Plus Student 108 Pointsr home
Courses Plus Student 108 PointsIt does work....but not for lowTemperature value...its always N/A for my app...any Idea why?