Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial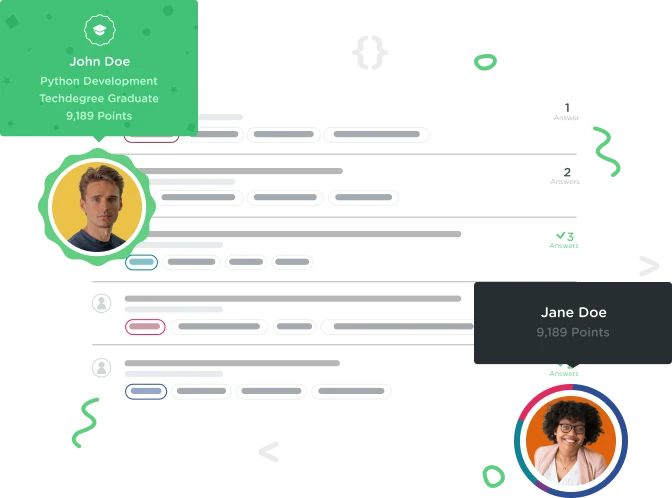
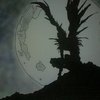
Lucas Santos
19,315 PointsWebpack dev server with ExpressJs
Ok so I have a very simple app with create-react-app. Now I'm using ExpressJS to handle API calls that I am making with the $.ajax() method inside of my react app within the componentWillMount() method.
So all the React front end has is a simple form with 1 input field and submit like so:
import React, { Component } from 'react';
import $ from 'jquery';
import './App.css';
class App extends Component {
componentDidMount(){
$('form').on('submit', function(e) {
e.preventDefault();
$.ajax({
url: '/api',
type: 'post',
data: $('form').serialize(),
dataType: 'json',
success: function(data) {
console.log('successful ajax request made!');
}
});
});
}
render() {
return (
<div className="App">
<form method="POST">
<input type="text" name="title" />
<input id="submit" type="submit" />
</form>
</div>
);
}
}
export default App;
Now my express route look like this:
var express = require("express");
var router = express.Router();
router.post("/api", function(req, res, next){
console.log(req.body);
});
module.exports = router;
THE PROBLEM
When running create-react-app with npm start it goes to localhost:3000 do to the Webpack local dev server and when I fire off my app.js in my server directory with node server/app.js is goes to localhost:3001 because they cannot run in the same port.
Because of this I cannot make proper API calls without getting an error showing that the POST request has failed.
So now the golden question is how can I make API calls from a local server on 2 different ports?
If you need any more info about my code i'd be more than happy to post it. Help is greatly appreciated, thank you!
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsYou need to enable CORS to do this.
See this answer to a similar question on Stack Overflow, specifically the Express part.