Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial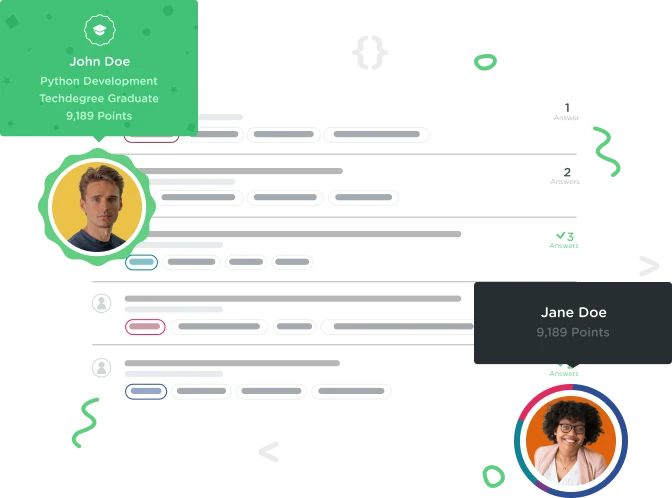
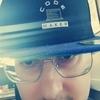
Magnus Martin
44,123 PointsWebpack Error using latest version 3.6.0
Hi,
when running npm run build webpack is transpiling with no error. But in Chrome developer tools I get the following error: "Uncaught TypeError: (0 , _util2.default) is not a function". What is going wrong? Any help is highly appreciated.
webpack.config.js looks like the following:
const webpack = require('webpack'),
UglifyJSPlugin = require('uglifyjs-webpack-plugin'),
WebpackBabelExternalsPlugin = require('webpack-babel-external-helpers-2'),
path = require('path');
let webpackConfig = {
entry: [
"./src/js/vendor/index.js",
"./src/js/app/index.js",
"./src/js/lib/index.js"
],
output: {
path: __dirname + "/src/js",
filename: "app.js"
},
module: {
rules: [
{
loader: "babel-loader",
exclude: [
path.resolve(__dirname, "node_modules")
],
test: /\.js$/,
}
]
},
plugins: [
new webpack.ProvidePlugin({
$: 'jquery',
jQuery: 'jquery',
'window.jQuery': 'jquery',
// In case you imported plugins individually, you must also require them here:
Util: "exports-loader?Util!bootstrap/js/dist/util",
Dropdown: "exports-loader?Dropdown!bootstrap/js/dist/dropdown",
Tab: "exports-loader?Tab!bootstrap/js/dist/tab",
}),
new UglifyJSPlugin({
compress: false,
mangle: false,
beautify: true,
comments: true,
warnings: false,
sourceMap: true
}),
new WebpackBabelExternalsPlugin()
]
};
module.exports = webpackConfig;
And my package.json dependencies look like this:
"dependencies": {
"babel-cli": "^6.26.0",
"babel-core": "^6.26.0",
"babel-loader": "^7.1.2",
"babel-preset-env": "^1.6.0",
"bootstrap": "^4.0.0-alpha.6",
"css-loader": "^0.28.7",
"exports-loader": "^0.6.4",
"html-webpack-plugin": "^2.30.1",
"jquery": "^3.2.1",
"list.js": "^1.5.0",
"mustache": "^2.3.0",
"node-sass": "^4.5.3",
"popper.js": "^1.12.5",
"sass-loader": "^6.0.6",
"style-loader": "^0.18.2",
"tether": "^1.4.0",
"uglify-es": "^3.1.2",
"uglifyjs-webpack-plugin": "^0.4.6",
"webpack": "^3.6.0",
"webpack-babel-external-helpers-2": "^2.0.0",
"webpack-dev-server": "^2.9.1"
}
1 Answer
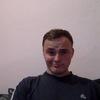
Ivan Baranov
11,344 PointsI'm not a webpack pro, but your config looks a bit odd. Try following official guide https://webpack.js.org/guides/. also this guide would be pretty helpful, even though it's about webpack 2: https://www.youtube.com/watch?v=JdGnYNtuEtE&list=PLkEZWD8wbltnRp6nRR8kv97RbpcUdNawY Here is my webpack config: webpack.config.js/
const path = require('path');
const webpack = require('webpack');
const HtmlWebpackPlugin = require('html-webpack-plugin');
const CleanWebpackPlugin = require('clean-webpack-plugin');
module.exports = {
entry: {
app: './src/app.js',
slider: './src/slider.js'
},
devtool: "source-map",
devServer: {
historyApiFallback: true,
contentBase: path.join(__dirname, "build")
},
plugins: [
new CleanWebpackPlugin(['build']),
new HtmlWebpackPlugin({
title: 'Output Management',
minify: {
collapseWhitespace: true
},
template: './src/index.html'
})
],
output: {
filename: '[name].bundle.js',
path: path.resolve(__dirname, 'build')
},
module:{
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
loader: 'babel-loader',
options: {
presets: ['react', 'es2015', 'stage-1']
}
},
{
test: /\.sass$/,
use: [{
loader: "style-loader"
}, {
loader: "css-loader", options: {
sourceMap: true
}
},{
loader: "sass-loader", options: {
sourceMap: true
}
}]
},
{
test: /\.(gif|png|jpg|svg)$/i,
use: [
'file-loader?name=[name].[ext]&outputPath=images/',
'image-webpack-loader'
]
},
{
test: /\.(woff|woff2|eot|ttf|otf)$/,
use: [
'file-loader'
]
},
{
test: /\.(csv|tsv)$/,
use: [
'csv-loader'
]
},
{
test: /\.xml$/,
use: [
'xml-loader'
]
}
//end
]
}
};
webpack.config.dev.js/
const config = require('./webpack.config.js');
const path = require('path');
const webpack = require('webpack');
const merge = require('webpack-merge');
const CleanWebpackPlugin = require('clean-webpack-plugin');
module.exports = merge(config, {
devtool: "source-map",
devServer: {
historyApiFallback: true,
contentBase: path.join(__dirname, "build")
},
plugins: [
new webpack.HotModuleReplacementPlugin(),
]
});
webpack.config.prod.js/
const webpack = require('webpack');
const merge = require('webpack-merge');
const config = require('./webpack.config.js');
const ExtractTextPlugin = require("extract-text-webpack-plugin");
const CompressionPlugin = require("compression-webpack-plugin");
const UglifyJSPlugin = require('uglifyjs-webpack-plugin');
const OptimizeCssAssetsPlugin = require('optimize-css-assets-webpack-plugin');
module.exports = merge(config, {
devtool: 'source-map',
plugins: [
new UglifyJSPlugin({
sourceMap: true
}),
new CompressionPlugin({
asset: "[path].gz[query]",
algorithm: "gzip",
test: /\.(js|html|cssbuild)$/,
threshold: 10240,
minRatio: 0.8
}),
new ExtractTextPlugin({
filename: 'main.css',
allChunks: true,
}),
new webpack.DefinePlugin({
'process.env':{
'NODE_ENV': JSON.stringify('production')
}
}),
new OptimizeCssAssetsPlugin({
assetNameRegExp: /\.css$/g,
cssProcessor: require('cssnano'),
cssProcessorOptions: { discardComments: {removeAll: true}},
canPrint: true
})
],
module: {
rules: [
{
test: /\.sass$/,
use: ExtractTextPlugin.extract({
fallback: 'style-loader',
use: [{
loader: 'css-loader',
},
{
loader: 'sass-loader'
}]
})
}
]
}
});