Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial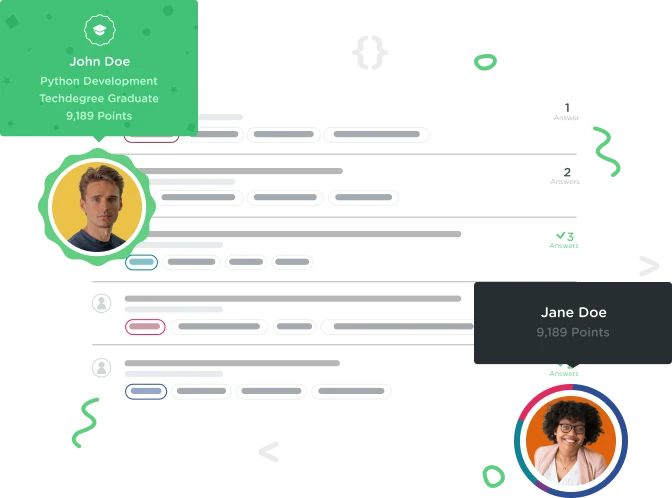

rachelweaver
15,876 PointsWebsite Security?
I am about to FTP a website and I have wondered are there any security bases I need to cover? It is a basic portfolio site(no sign up or e-commerce). I do have my contact info on here though and I want to protect myself from spammers and viruses. Too often I have stumbled across a website only for it to infect my computer or have anti-virus alerts go wild. I don't want my site to be one of those websites. Any suggestions?
3 Answers
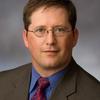
Ted Sumner
Courses Plus Student 17,967 PointsThis is the code from the Treehouse PHP course to protect against getting your site hijacked.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// the form has fields for name, email, and message
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$message = trim($_POST["message"]);
// the fields name, email, and message are required
if ($name == "" OR $email == "" OR $message == "") {
$error_message = "You must specify a value for name, email address, and message.";
}
// this code checks for malicious code attempting
// to inject values into the email header
if (!isset($error_message)) {
foreach( $_POST as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "There was a problem with the information you entered.";
}
}
}
// the field named address is used as a spam honeypot
// it is hidden from users, and it must be left blank
if (!isset($error_message) && $_POST["address"] != "") {
$error_message = "Your form submission has an error.";
}
The first step is to trim the input. Trim removes white space from the beginning and end of the input. . It prevents a space from being viewed as a valid entry.
After the trim, the code is checked for all mandatory fields.
The stripos function strips all the symbols that create code out of the entry, protecting against code inserts. This is extra important if you display user entries back to the site.
The next block is a honeypot like described above. It is hidden by CSS and there is a label saying that humans should leave it blank in case it shows up.
There are issues presented by this stuff that I never had a clue of before these courses.

Brandon Bolin
760 PointsA basic HTML/CSS website has little risk of infection from malware (although still possible). Most common is contact form script injection so be sure to use proper human checking methods in your forms.
There are different versions of FTP. There's FTP and then sFTP which is more secure. Again, given that there is no confidential info on a simple portfolio website, FTP would be fine (as far as I'm aware).
Some hosts have add-ons such as SiteLock Security that watches your server and will notify you if someone has uploaded a file (that wasn't yourself) and will scan for malicious files IF your website is ever actually compromised.
Hope that helps somewhat...

rachelweaver
15,876 PointsWhat about jQuery? Will that affect anyting?

Brandon Bolin
760 PointsjQuery in what manner? As far as I'm aware, there isn't much of a security issue with "basic" jQuery stuff, like hiding/showing divs, animating, etc.
There are methods of using javascript/jQuery to display email addresses so that it's not in the HTML (gets less spam)
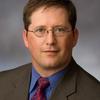
Ted Sumner
Courses Plus Student 17,967 PointsThe real issue you will have is spammers if you have your email address on the site. I have seen people spell it out. I put in a contact form, but no email on my site. I advise against email address on sites. I have an email that gets 50 spam a day because I had it on a site for about 2 months in 2009.

rachelweaver
15,876 PointsThanks, I held off on FTP until I get that sorted out.

Brandon Bolin
760 PointsYou might also want to explore Javascript/jQuery to display email addresses - but yes, certainly use a contact form.
Note, that contact forms themselves are not secure at all! I had a basic HTML/PHP form and got so much spam that my server was shutdown because I was hitting outgoing email limits (literally hundreds an hour).
If you are using WordPress with this, make sure you enable Akismet and use Contact Form 7, or Gravity Forms.
If you are using just HTML, I based my form off of this one: https://css-tricks.com/nice-and-simple-contact-form/
Then you simply need to add some extra anti-bot checks. One common one is a math question. So create a new input field on the form called math or something. Then in the PHP file (you'll see and understand in that link), you surround the form validation with a PHP IF check.
Below is from my own form:
if($LastName == '' && $Spam == '5' && $Message !== ''){
(the rest of the form code in here)
}
$LastName is actually a fake value so that bots think they need to fill it in. If it contains content (it's a hidden input field so real users wouldn't fill it in), then the form won't submit.
$Spam is my math checker, so I just have input with label 2+3. If input answer is 5, then continue on.
$Message !=='' checks to make sure there is actually a message and again not a bot just trying to fill in name, and website.
Last, it's important to make sure bots are not embedding malicious scripts into the input fields. Essentially you use preg_match php function for that (my example below):
if ( preg_match( "/[\r\n]/", $Name ) || preg_match( "/[\r\n]/", $Email ) ) {
print "<meta http-equiv=\"refresh\" content=\"0;URL=contactthanks3.php\">";
}
My WHOLE contactengine.php from css-tricks.com (not saying it's 100% awesome, but it's what I managed to setup and it's stopped all spam since):
<?php
$EmailFrom = "email@email.com";
$EmailTo = "email@email.com";
$Subject = "New Website Inquiry";
$Name = Trim(stripslashes($_POST['Name']));
$LastName = Trim(stripslashes($_POST['last_name']));
$Spam = $_POST['Spam'];
$Phone = Trim(stripslashes($_POST['Phone']));
$Email = Trim(stripslashes($_POST['Email']));
$Message = Trim(stripslashes($_POST['Message']));
if ( preg_match( "/[\r\n]/", $Name ) || preg_match( "/[\r\n]/", $Email ) ) {
print "<meta http-equiv=\"refresh\" content=\"0;URL=contactthanks3.php\">";
}
if($LastName == '' && $Spam == '5' && $Message !== ''){
// validation
$validationOK=true;
if (!$validationOK) {
print "<meta http-equiv=\"refresh\" content=\"0;URL=error1.php\">";
exit;
}
// prepare email body text
$Body = "";
$Body .= "Name: ";
$Body .= $Name;
$Body .= "\n";
$Body .= "Phone: ";
$Body .= $Phone;
$Body .= "\n";
$Body .= "Email: ";
$Body .= $Email;
$Body .= "\n";
$Body .= "Message: ";
$Body .= $Message;
$Body .= "\n";
//send email
$success = mail($EmailTo, $Subject, $Body, "From: <$EmailFrom>");
//redirect to success page
if ($success){
print "<meta http-equiv=\"refresh\" content=\"0;URL=contactthanks.php\">";
exit;
}
else{
print "<meta http-equiv=\"refresh\" content=\"0;URL=error2.php\">";
}
} print "<meta http-equiv=\"refresh\" content=\"0;URL=contactthanks2.php\">";
?>
rachelweaver
15,876 Pointsrachelweaver
15,876 PointsThanks! I will look at this!
rachelweaver
15,876 Pointsrachelweaver
15,876 PointsThanks, this helped me quite a bit!
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 PointsYou are welcome.