Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial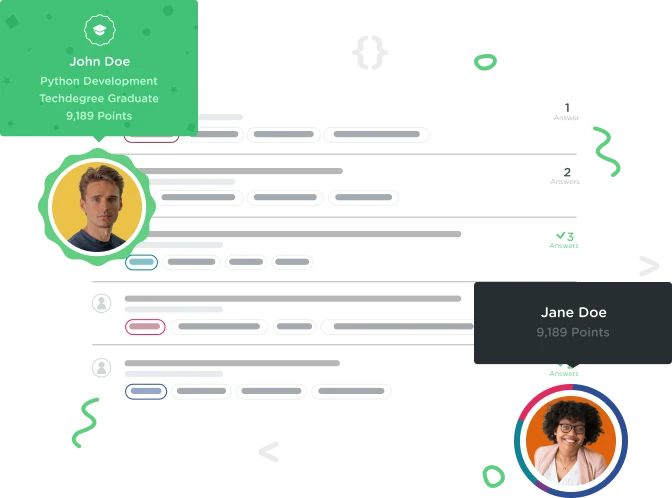

Khaleel Yusuf
15,208 PointsWe're playing a popular board game about snatching up real estate in Atlantic City. I need you finish out the Capitalism
I need so much help.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self, *args, **kwargs):
super().__init__(size=2, die_class=D6, *args, **kwargs)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
11 Answers
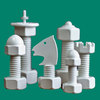
Steven Parker
231,269 PointsBut the point is to learn how to do it.
So the instructions say, "Alright! Now I need you to add a new property called doubles. It should return True if both of the dice have the same value. Otherwise, return False." Let's try breaking it down to smaller steps:
- add a "property" line
- add a "def" line to declare the method
- the name of the method is "doubles"
- the method takes no arguments
- the object has 2 dice in it (you made that happen in task 1)
- each item can be accessed by indexing ("
self[0]
" and "self[1]
") - an equality comparison ("==") will return True of both items have the same value
I'll bet you can do it now.

Khaleel Yusuf
15,208 PointsEverything.
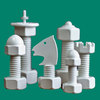
Steven Parker
231,269 PointsThat's not exactly "more specific". But try breaking the instructions down into smaller steps and/or re-watching the videos.

Khaleel Yusuf
15,208 PointsI did rewatch the videos and I didn't understand anything. Could just give me the code to finish this code challenge, please?

Khaleel Yusuf
15,208 PointsI don't understand
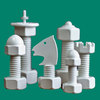
Steven Parker
231,269 PointsCan you be a bit more specific? I'm not sure what part you are having trouble with.
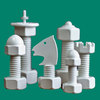
Steven Parker
231,269 PointsIt looks like you've passed task 1 but have not yet done task 2.
For task 2 you will " add a new property called doubles". There are a lot of properties already to serve as a model for the basic structure of a property. Then, your property "should return True
if both of the dice have the same value". So since "CapitalismHand" is a type of "list:", you can directly return the result of comparing the first two items (the ones with index values "0" and "1") with each other.
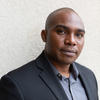
pierreilyamukuru
9,831 Pointsclass CapitalismHand(Hand): def init(self,*args, **kwargs): super().init(size=2, die_class=D6, *args, **kwargs) @property def ones(self): return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}

Khaleel Yusuf
15,208 PointsIsn't this correct: @property def doubles(self): if self[0] == self[1]: return True
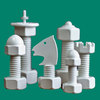
Steven Parker
231,269 PointsVery close! Now you just need to return False if the values don't match.
To show code with indentation, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Khaleel Yusuf
15,208 PointsMy code won't let me pass the first question.
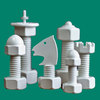
Steven Parker
231,269 PointsShow the code as it is now. Be sure to format it.

Khaleel Yusuf
15,208 Pointsfrom dice import D6
class Hand(list):
def init(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().init()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def init(self, args, **kwargs):
super().init(size=2, die_class=D6, *args, *kwargs)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}

Khaleel Yusuf
15,208 PointsI indented it but it didn't work
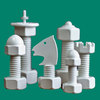
Steven Parker
231,269 PointsThe formatting seems to be correct, which would mean that the indentation is not correct.
Also, the task 1 code which was correct before seems to have changed and is no longer good. Is used to have "__init__
" but now only has "init
".
And I can't see the code you added for "doubles".

Khaleel Yusuf
15,208 PointsNow I need help on the next question: And, finally, if I have doubles, I want to reroll the hand. Add a classmethod to CapitalismHand named reroll that returns a new instance of the class, effectively rerolling the hand.
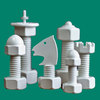
Steven Parker
231,269 PointsThe next step is just a matter of declaring a "classmethod", and creating a new "Hand" object in it and returning it. If you still have trouble, start a new question which includes your code.
Happy coding!
Omar G.
3,620 PointsOmar G.
3,620 PointsThanks for this explanation. I was trying to do something like self.value == self. value but as soon as I read "the object has 2 dice in it (you made that happen in task 1)" I understood how to solve this problem