Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial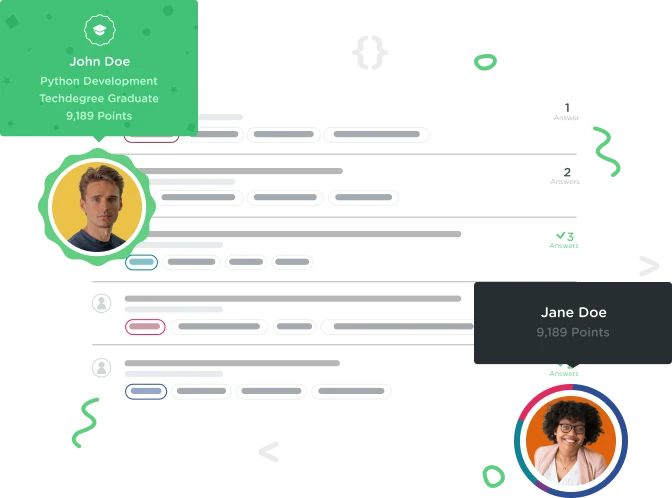

Karen Shumate
13,579 PointsWe're playing a popular board game about snatching up real estate in Atlantic City. I need you finish out the Capitalism
Need help, tell me what I'm doing wrong.
Thanks,
Karen
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self, *args, **kwargs):
super().__init__(die=2, die_class=D6, *args, **kwargs)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
1 Answer
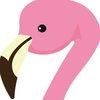
Dave StSomeWhere
19,870 PointsI see two things incorrect.
1 - the indentation on def init 2 - the argument should be size not die (based on the Hand init)
Should look like:
class CapitalismHand(Hand):
# you are a space off below (should line up with the @property below)
# size instead of die
def __init__(self, *args, **kwargs):
super().__init__(size=2, die_class=D6, *args, **kwargs)
@property
def ones(self):
return self._by_value(1)