Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial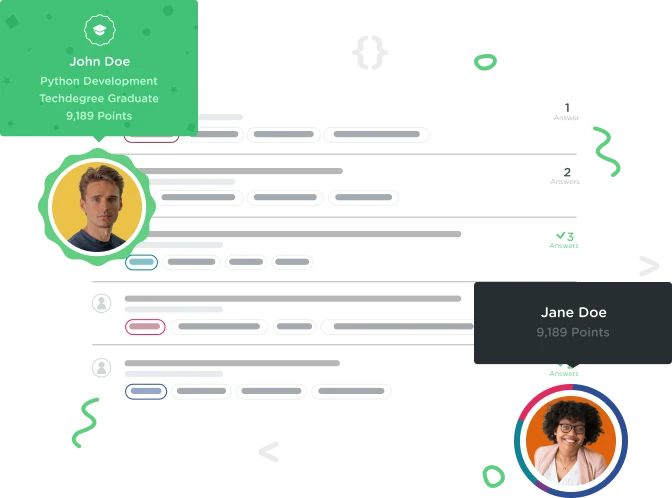

David Regel
Full Stack JavaScript Techdegree Student 5,504 PointsWh does my while loop stops looping?
My JavaScript File looks like this:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search student records: type a name [Jody] (or type 'quit' to end)");
if (search.toLowerCase = "quit") {
break;
}
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}
When I type "quit" in the prompt window, my loop will stop working. This is correct. However, even when I type something else in the prompt window, my loop will also stop working. My goal is that the prompt window will appear as long as the user types something else than "quit".
Can someone tell me what's wrong with my code?
1 Answer

andren
28,558 PointsThe reason it always quits is that you are using the = operator instead of the == operator within the if
condition. = is used to assign a value, == is used to compare values.
There is another issue though, which is the fact that you have not put parenthesis after your toLowerCase
method call. In JavaScript you always have to put a pair of parenthesis after a method name in order to actually run it.
If you fix those issues like this:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search student records: type a name [Jody] (or type 'quit' to end)");
if (search.toLowerCase() == "quit") { // Added () and changed = to ==
break;
}
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}
Then your prompt code will work properly.
David Regel
Full Stack JavaScript Techdegree Student 5,504 PointsDavid Regel
Full Stack JavaScript Techdegree Student 5,504 PointsYou are AWESOME! :) Thanks